A function which translates a sentence to title-caseTitle case name when first, middle and last are all upper or lower caseCounting Words in Files - MATLAB styleFinding the first non-repeating character in a stringCounting words, letters, average word length, and letter frequencyTitle case a sentence functionTest if a string is a palindromeLeetcode 49: Group Anagrams - Hash function design talkAbbreviate a string using dictionary in CTitle Case ConverterGeneric Case Converter
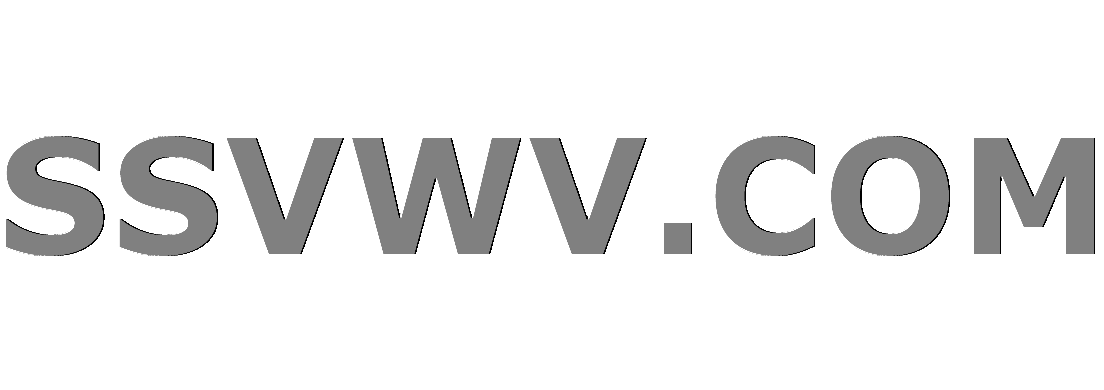
Multi tool use
A function which translates a sentence to title-case
Show that if two triangles built on parallel lines, with equal bases have the same perimeter only if they are congruent.
Why does Kotter return in Welcome Back Kotter?
If I cast Expeditious Retreat, can I Dash as a bonus action on the same turn?
"You are your self first supporter", a more proper way to say it
"which" command doesn't work / path of Safari?
strToHex ( string to its hex representation as string)
Why not use SQL instead of GraphQL?
Shell script not opening as desktop application
Mains transformer blew up amplifier, incorrect description in wiring instructions?
Why don't electromagnetic waves interact with each other?
Relation between Frobenius, spectral norm and sum of maxima
Japan - Plan around max visa duration
Draw simple lines in Inkscape
What would happen to a modern skyscraper if it rains micro blackholes?
If two metric spaces are topologically equivalent (homeomorphic) imply that they are complete?
Methods for deciding between [odd number] players
What typically incentivizes a professor to change jobs to a lower ranking university?
Is it legal for company to use my work email to pretend I still work there?
Is it tax fraud for an individual to declare non-taxable revenue as taxable income? (US tax laws)
Writing rule which states that two causes for the same superpower is bad writing
Why don't electron-positron collisions release infinite energy?
How to add power-LED to my small amplifier?
Can I interfere when another PC is about to be attacked?
A function which translates a sentence to title-case
Title case name when first, middle and last are all upper or lower caseCounting Words in Files - MATLAB styleFinding the first non-repeating character in a stringCounting words, letters, average word length, and letter frequencyTitle case a sentence functionTest if a string is a palindromeLeetcode 49: Group Anagrams - Hash function design talkAbbreviate a string using dictionary in CTitle Case ConverterGeneric Case Converter
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty margin-bottom:0;
$begingroup$
The task is to write a JavaScript-function which translates a given blank-separated sentence to title-case.
Means that all words shall start with a capital and then the rest of the word in lower-case. But: A certain, specified set of conjunctions, preposition as well as article shall be all lower-case.
Example: "The second of the four items." becomes "The Second of the Four Items.".
Here's my implementation of such a function:
function translateToTitleCase(str)
const translateWord = (sWord) =>
return sWord.slice(0, 1).toUpperCase() + sWord.slice(1).toLowerCase();
const words = str.split(" ");
words[0] = translateWord(words[0]);
for (let i = 1; i < words.length; i++)
if (!["of", "and", "the", "to"].includes(words[i].toLowerCase()))
words[i] = translateWord(words[i]);
else
words[i] = words[i].toLowerCase(); // Make sure is's the correct case, when the sentence (or parts of it) is given in uppercase.
return words.join(" ");
// -- Examples -------------------------------------------------------------------------------
console.log(translateToTitleCase("Into unmerciful the entreating stronger to of word guessing."));
console.log(translateToTitleCase("the OLD MAN aND THE sEa"));
I think my coding is still a bit "noisy" with the usage of all those brackets, chained methods and concatenation.
Any ideas about how to improve my implementation?
Perhaps some cool new ES6-feature I wasn't aware of.
What would you have done differently and why?
javascript strings
$endgroup$
add a comment |
$begingroup$
The task is to write a JavaScript-function which translates a given blank-separated sentence to title-case.
Means that all words shall start with a capital and then the rest of the word in lower-case. But: A certain, specified set of conjunctions, preposition as well as article shall be all lower-case.
Example: "The second of the four items." becomes "The Second of the Four Items.".
Here's my implementation of such a function:
function translateToTitleCase(str)
const translateWord = (sWord) =>
return sWord.slice(0, 1).toUpperCase() + sWord.slice(1).toLowerCase();
const words = str.split(" ");
words[0] = translateWord(words[0]);
for (let i = 1; i < words.length; i++)
if (!["of", "and", "the", "to"].includes(words[i].toLowerCase()))
words[i] = translateWord(words[i]);
else
words[i] = words[i].toLowerCase(); // Make sure is's the correct case, when the sentence (or parts of it) is given in uppercase.
return words.join(" ");
// -- Examples -------------------------------------------------------------------------------
console.log(translateToTitleCase("Into unmerciful the entreating stronger to of word guessing."));
console.log(translateToTitleCase("the OLD MAN aND THE sEa"));
I think my coding is still a bit "noisy" with the usage of all those brackets, chained methods and concatenation.
Any ideas about how to improve my implementation?
Perhaps some cool new ES6-feature I wasn't aware of.
What would you have done differently and why?
javascript strings
$endgroup$
$begingroup$
I'd argue that the functionality should be changed slightly – this'll trip over words like xkcd and eBay – but I suppose that's not really in-scope for Code Review.
$endgroup$
– wizzwizz4
47 mins ago
add a comment |
$begingroup$
The task is to write a JavaScript-function which translates a given blank-separated sentence to title-case.
Means that all words shall start with a capital and then the rest of the word in lower-case. But: A certain, specified set of conjunctions, preposition as well as article shall be all lower-case.
Example: "The second of the four items." becomes "The Second of the Four Items.".
Here's my implementation of such a function:
function translateToTitleCase(str)
const translateWord = (sWord) =>
return sWord.slice(0, 1).toUpperCase() + sWord.slice(1).toLowerCase();
const words = str.split(" ");
words[0] = translateWord(words[0]);
for (let i = 1; i < words.length; i++)
if (!["of", "and", "the", "to"].includes(words[i].toLowerCase()))
words[i] = translateWord(words[i]);
else
words[i] = words[i].toLowerCase(); // Make sure is's the correct case, when the sentence (or parts of it) is given in uppercase.
return words.join(" ");
// -- Examples -------------------------------------------------------------------------------
console.log(translateToTitleCase("Into unmerciful the entreating stronger to of word guessing."));
console.log(translateToTitleCase("the OLD MAN aND THE sEa"));
I think my coding is still a bit "noisy" with the usage of all those brackets, chained methods and concatenation.
Any ideas about how to improve my implementation?
Perhaps some cool new ES6-feature I wasn't aware of.
What would you have done differently and why?
javascript strings
$endgroup$
The task is to write a JavaScript-function which translates a given blank-separated sentence to title-case.
Means that all words shall start with a capital and then the rest of the word in lower-case. But: A certain, specified set of conjunctions, preposition as well as article shall be all lower-case.
Example: "The second of the four items." becomes "The Second of the Four Items.".
Here's my implementation of such a function:
function translateToTitleCase(str)
const translateWord = (sWord) =>
return sWord.slice(0, 1).toUpperCase() + sWord.slice(1).toLowerCase();
const words = str.split(" ");
words[0] = translateWord(words[0]);
for (let i = 1; i < words.length; i++)
if (!["of", "and", "the", "to"].includes(words[i].toLowerCase()))
words[i] = translateWord(words[i]);
else
words[i] = words[i].toLowerCase(); // Make sure is's the correct case, when the sentence (or parts of it) is given in uppercase.
return words.join(" ");
// -- Examples -------------------------------------------------------------------------------
console.log(translateToTitleCase("Into unmerciful the entreating stronger to of word guessing."));
console.log(translateToTitleCase("the OLD MAN aND THE sEa"));
I think my coding is still a bit "noisy" with the usage of all those brackets, chained methods and concatenation.
Any ideas about how to improve my implementation?
Perhaps some cool new ES6-feature I wasn't aware of.
What would you have done differently and why?
function translateToTitleCase(str)
const translateWord = (sWord) =>
return sWord.slice(0, 1).toUpperCase() + sWord.slice(1).toLowerCase();
const words = str.split(" ");
words[0] = translateWord(words[0]);
for (let i = 1; i < words.length; i++)
if (!["of", "and", "the", "to"].includes(words[i].toLowerCase()))
words[i] = translateWord(words[i]);
else
words[i] = words[i].toLowerCase(); // Make sure is's the correct case, when the sentence (or parts of it) is given in uppercase.
return words.join(" ");
// -- Examples -------------------------------------------------------------------------------
console.log(translateToTitleCase("Into unmerciful the entreating stronger to of word guessing."));
console.log(translateToTitleCase("the OLD MAN aND THE sEa"));
function translateToTitleCase(str)
const translateWord = (sWord) =>
return sWord.slice(0, 1).toUpperCase() + sWord.slice(1).toLowerCase();
const words = str.split(" ");
words[0] = translateWord(words[0]);
for (let i = 1; i < words.length; i++)
if (!["of", "and", "the", "to"].includes(words[i].toLowerCase()))
words[i] = translateWord(words[i]);
else
words[i] = words[i].toLowerCase(); // Make sure is's the correct case, when the sentence (or parts of it) is given in uppercase.
return words.join(" ");
// -- Examples -------------------------------------------------------------------------------
console.log(translateToTitleCase("Into unmerciful the entreating stronger to of word guessing."));
console.log(translateToTitleCase("the OLD MAN aND THE sEa"));
javascript strings
javascript strings
edited 6 hours ago
michael.zech
asked 6 hours ago
michael.zechmichael.zech
1,7631735
1,7631735
$begingroup$
I'd argue that the functionality should be changed slightly – this'll trip over words like xkcd and eBay – but I suppose that's not really in-scope for Code Review.
$endgroup$
– wizzwizz4
47 mins ago
add a comment |
$begingroup$
I'd argue that the functionality should be changed slightly – this'll trip over words like xkcd and eBay – but I suppose that's not really in-scope for Code Review.
$endgroup$
– wizzwizz4
47 mins ago
$begingroup$
I'd argue that the functionality should be changed slightly – this'll trip over words like xkcd and eBay – but I suppose that's not really in-scope for Code Review.
$endgroup$
– wizzwizz4
47 mins ago
$begingroup$
I'd argue that the functionality should be changed slightly – this'll trip over words like xkcd and eBay – but I suppose that's not really in-scope for Code Review.
$endgroup$
– wizzwizz4
47 mins ago
add a comment |
1 Answer
1
active
oldest
votes
$begingroup$
I find this code quite reasonable on the whole, but you might consider the following suggestions relating to consistency, succinctness and semantics.
Move and rename the inner helper function
translateWord
is reusable as a general utility function and seems better-placed in the global scope and renamed to titleCase
. This meshes with similarly-named Ruby, Python and PHP builtins (titlecase
, title
, ucfirst
, respectively). When I see "translate", I think of linguistics or mathematics before I think of strings or casing.
Excessive calls to toLowerCase
.toLowerCase()
is more efficient called once on the entire sentence before splitting rather than incurring the overhead of calling it multiple times per word. With this in mind, you can skip calling the titleCase
function described above if you wish.
Improve "ignore" list
if (!["of", "and", "the", "to"].includes(words[i].toLowerCase())) {
is problematic for a few reasons:
- It creates a new array object for every word. Move initialization to the top of the function and create it once.
Hardcoding restricts your function's reusability. Making this ignore list a default parameter allows the client to adjust the list as needed.- Giving this array a variable name makes its purpose more obvious.
- Although the array is small, it needs to be traversed linearly to perform a lookup; using a set improves semantics, readability and time complexity all at once and is the ideal structure for testing membership.
Avoid the loop
This task is a map operation: each word has a function applied to it. You can roll split
, map
(your for
loop) and join
into one call to replace
, which takes a regular expression that splits on non-word characters and applies the titleCase
function to each one that passes the ignore
test.
Minor points
sWord.slice(0, 1)
can besWord[0]
.sWord
is an okay variable name, butstr
(matching your outer function) orword
seems more consistent.- Unless there is a good hoisting or context reason, I'd make the outer function also use an arrow function for consistency with your inner function.
A rewrite
const titleCaseWords = (words, ignore=["of", "and", "the", "to"]) =>
ignore = new Set(ignore);
return words.replace(/w+/g, (word, i) =>
word = word.toLowerCase();
if (i && ignore.has(word))
return word;
return word[0].toUpperCase() + word.slice(1);
);
;
[
"Into unmerciful the entreating stronger to of word guessing.",
"the OLD MAN aND THE sEa"
].forEach(test => console.log(titleCaseWords(test)));
$endgroup$
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
return StackExchange.using("mathjaxEditing", function ()
StackExchange.MarkdownEditor.creationCallbacks.add(function (editor, postfix)
StackExchange.mathjaxEditing.prepareWmdForMathJax(editor, postfix, [["\$", "\$"]]);
);
);
, "mathjax-editing");
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "196"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f217006%2fa-function-which-translates-a-sentence-to-title-case%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
$begingroup$
I find this code quite reasonable on the whole, but you might consider the following suggestions relating to consistency, succinctness and semantics.
Move and rename the inner helper function
translateWord
is reusable as a general utility function and seems better-placed in the global scope and renamed to titleCase
. This meshes with similarly-named Ruby, Python and PHP builtins (titlecase
, title
, ucfirst
, respectively). When I see "translate", I think of linguistics or mathematics before I think of strings or casing.
Excessive calls to toLowerCase
.toLowerCase()
is more efficient called once on the entire sentence before splitting rather than incurring the overhead of calling it multiple times per word. With this in mind, you can skip calling the titleCase
function described above if you wish.
Improve "ignore" list
if (!["of", "and", "the", "to"].includes(words[i].toLowerCase())) {
is problematic for a few reasons:
- It creates a new array object for every word. Move initialization to the top of the function and create it once.
Hardcoding restricts your function's reusability. Making this ignore list a default parameter allows the client to adjust the list as needed.- Giving this array a variable name makes its purpose more obvious.
- Although the array is small, it needs to be traversed linearly to perform a lookup; using a set improves semantics, readability and time complexity all at once and is the ideal structure for testing membership.
Avoid the loop
This task is a map operation: each word has a function applied to it. You can roll split
, map
(your for
loop) and join
into one call to replace
, which takes a regular expression that splits on non-word characters and applies the titleCase
function to each one that passes the ignore
test.
Minor points
sWord.slice(0, 1)
can besWord[0]
.sWord
is an okay variable name, butstr
(matching your outer function) orword
seems more consistent.- Unless there is a good hoisting or context reason, I'd make the outer function also use an arrow function for consistency with your inner function.
A rewrite
const titleCaseWords = (words, ignore=["of", "and", "the", "to"]) =>
ignore = new Set(ignore);
return words.replace(/w+/g, (word, i) =>
word = word.toLowerCase();
if (i && ignore.has(word))
return word;
return word[0].toUpperCase() + word.slice(1);
);
;
[
"Into unmerciful the entreating stronger to of word guessing.",
"the OLD MAN aND THE sEa"
].forEach(test => console.log(titleCaseWords(test)));
$endgroup$
add a comment |
$begingroup$
I find this code quite reasonable on the whole, but you might consider the following suggestions relating to consistency, succinctness and semantics.
Move and rename the inner helper function
translateWord
is reusable as a general utility function and seems better-placed in the global scope and renamed to titleCase
. This meshes with similarly-named Ruby, Python and PHP builtins (titlecase
, title
, ucfirst
, respectively). When I see "translate", I think of linguistics or mathematics before I think of strings or casing.
Excessive calls to toLowerCase
.toLowerCase()
is more efficient called once on the entire sentence before splitting rather than incurring the overhead of calling it multiple times per word. With this in mind, you can skip calling the titleCase
function described above if you wish.
Improve "ignore" list
if (!["of", "and", "the", "to"].includes(words[i].toLowerCase())) {
is problematic for a few reasons:
- It creates a new array object for every word. Move initialization to the top of the function and create it once.
Hardcoding restricts your function's reusability. Making this ignore list a default parameter allows the client to adjust the list as needed.- Giving this array a variable name makes its purpose more obvious.
- Although the array is small, it needs to be traversed linearly to perform a lookup; using a set improves semantics, readability and time complexity all at once and is the ideal structure for testing membership.
Avoid the loop
This task is a map operation: each word has a function applied to it. You can roll split
, map
(your for
loop) and join
into one call to replace
, which takes a regular expression that splits on non-word characters and applies the titleCase
function to each one that passes the ignore
test.
Minor points
sWord.slice(0, 1)
can besWord[0]
.sWord
is an okay variable name, butstr
(matching your outer function) orword
seems more consistent.- Unless there is a good hoisting or context reason, I'd make the outer function also use an arrow function for consistency with your inner function.
A rewrite
const titleCaseWords = (words, ignore=["of", "and", "the", "to"]) =>
ignore = new Set(ignore);
return words.replace(/w+/g, (word, i) =>
word = word.toLowerCase();
if (i && ignore.has(word))
return word;
return word[0].toUpperCase() + word.slice(1);
);
;
[
"Into unmerciful the entreating stronger to of word guessing.",
"the OLD MAN aND THE sEa"
].forEach(test => console.log(titleCaseWords(test)));
$endgroup$
add a comment |
$begingroup$
I find this code quite reasonable on the whole, but you might consider the following suggestions relating to consistency, succinctness and semantics.
Move and rename the inner helper function
translateWord
is reusable as a general utility function and seems better-placed in the global scope and renamed to titleCase
. This meshes with similarly-named Ruby, Python and PHP builtins (titlecase
, title
, ucfirst
, respectively). When I see "translate", I think of linguistics or mathematics before I think of strings or casing.
Excessive calls to toLowerCase
.toLowerCase()
is more efficient called once on the entire sentence before splitting rather than incurring the overhead of calling it multiple times per word. With this in mind, you can skip calling the titleCase
function described above if you wish.
Improve "ignore" list
if (!["of", "and", "the", "to"].includes(words[i].toLowerCase())) {
is problematic for a few reasons:
- It creates a new array object for every word. Move initialization to the top of the function and create it once.
Hardcoding restricts your function's reusability. Making this ignore list a default parameter allows the client to adjust the list as needed.- Giving this array a variable name makes its purpose more obvious.
- Although the array is small, it needs to be traversed linearly to perform a lookup; using a set improves semantics, readability and time complexity all at once and is the ideal structure for testing membership.
Avoid the loop
This task is a map operation: each word has a function applied to it. You can roll split
, map
(your for
loop) and join
into one call to replace
, which takes a regular expression that splits on non-word characters and applies the titleCase
function to each one that passes the ignore
test.
Minor points
sWord.slice(0, 1)
can besWord[0]
.sWord
is an okay variable name, butstr
(matching your outer function) orword
seems more consistent.- Unless there is a good hoisting or context reason, I'd make the outer function also use an arrow function for consistency with your inner function.
A rewrite
const titleCaseWords = (words, ignore=["of", "and", "the", "to"]) =>
ignore = new Set(ignore);
return words.replace(/w+/g, (word, i) =>
word = word.toLowerCase();
if (i && ignore.has(word))
return word;
return word[0].toUpperCase() + word.slice(1);
);
;
[
"Into unmerciful the entreating stronger to of word guessing.",
"the OLD MAN aND THE sEa"
].forEach(test => console.log(titleCaseWords(test)));
$endgroup$
I find this code quite reasonable on the whole, but you might consider the following suggestions relating to consistency, succinctness and semantics.
Move and rename the inner helper function
translateWord
is reusable as a general utility function and seems better-placed in the global scope and renamed to titleCase
. This meshes with similarly-named Ruby, Python and PHP builtins (titlecase
, title
, ucfirst
, respectively). When I see "translate", I think of linguistics or mathematics before I think of strings or casing.
Excessive calls to toLowerCase
.toLowerCase()
is more efficient called once on the entire sentence before splitting rather than incurring the overhead of calling it multiple times per word. With this in mind, you can skip calling the titleCase
function described above if you wish.
Improve "ignore" list
if (!["of", "and", "the", "to"].includes(words[i].toLowerCase())) {
is problematic for a few reasons:
- It creates a new array object for every word. Move initialization to the top of the function and create it once.
Hardcoding restricts your function's reusability. Making this ignore list a default parameter allows the client to adjust the list as needed.- Giving this array a variable name makes its purpose more obvious.
- Although the array is small, it needs to be traversed linearly to perform a lookup; using a set improves semantics, readability and time complexity all at once and is the ideal structure for testing membership.
Avoid the loop
This task is a map operation: each word has a function applied to it. You can roll split
, map
(your for
loop) and join
into one call to replace
, which takes a regular expression that splits on non-word characters and applies the titleCase
function to each one that passes the ignore
test.
Minor points
sWord.slice(0, 1)
can besWord[0]
.sWord
is an okay variable name, butstr
(matching your outer function) orword
seems more consistent.- Unless there is a good hoisting or context reason, I'd make the outer function also use an arrow function for consistency with your inner function.
A rewrite
const titleCaseWords = (words, ignore=["of", "and", "the", "to"]) =>
ignore = new Set(ignore);
return words.replace(/w+/g, (word, i) =>
word = word.toLowerCase();
if (i && ignore.has(word))
return word;
return word[0].toUpperCase() + word.slice(1);
);
;
[
"Into unmerciful the entreating stronger to of word guessing.",
"the OLD MAN aND THE sEa"
].forEach(test => console.log(titleCaseWords(test)));
const titleCaseWords = (words, ignore=["of", "and", "the", "to"]) =>
ignore = new Set(ignore);
return words.replace(/w+/g, (word, i) =>
word = word.toLowerCase();
if (i && ignore.has(word))
return word;
return word[0].toUpperCase() + word.slice(1);
);
;
[
"Into unmerciful the entreating stronger to of word guessing.",
"the OLD MAN aND THE sEa"
].forEach(test => console.log(titleCaseWords(test)));
const titleCaseWords = (words, ignore=["of", "and", "the", "to"]) =>
ignore = new Set(ignore);
return words.replace(/w+/g, (word, i) =>
word = word.toLowerCase();
if (i && ignore.has(word))
return word;
return word[0].toUpperCase() + word.slice(1);
);
;
[
"Into unmerciful the entreating stronger to of word guessing.",
"the OLD MAN aND THE sEa"
].forEach(test => console.log(titleCaseWords(test)));
edited 2 hours ago
answered 2 hours ago
ggorlenggorlen
576213
576213
add a comment |
add a comment |
Thanks for contributing an answer to Code Review Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f217006%2fa-function-which-translates-a-sentence-to-title-case%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
zwys7dHHfQl W60SouC6f65Soi7h
$begingroup$
I'd argue that the functionality should be changed slightly – this'll trip over words like xkcd and eBay – but I suppose that's not really in-scope for Code Review.
$endgroup$
– wizzwizz4
47 mins ago