How can I improve readability and length of a method with many if statements?How can I concatenate two arrays in Java?How can I generate an MD5 hash?How can I pad an integer with zeros on the left?How can I initialise a static Map?How can I create an executable JAR with dependencies using Maven?How can I convert a stack trace to a string?How to make mock to void methods with MockitoHow to convert byte size into human readable format in java?How to reference a method in javadoc?Java sorting based on object one property with some condition
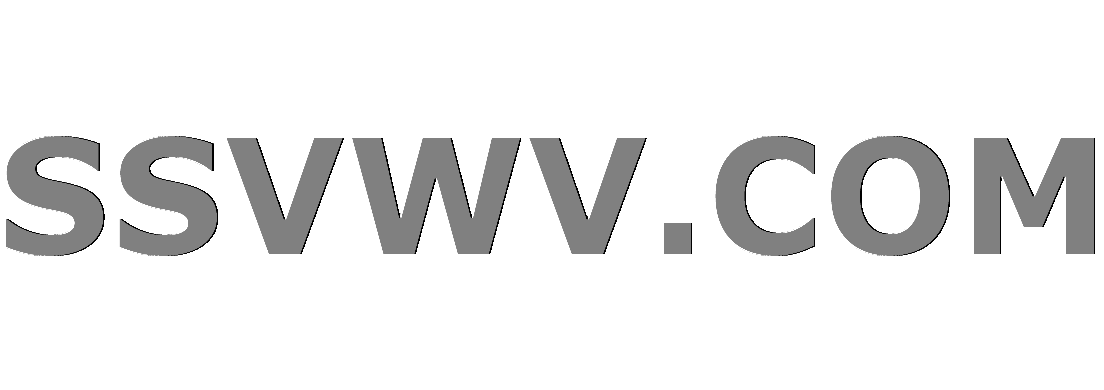
Multi tool use
Difference between "drift" and "wander"
Was the Lonely Mountain, where Smaug lived, a volcano?
I sent an angry e-mail to my interviewers about a conflict at my home institution. Could this affect my application?
Co-worker is now managing my team. Does this mean that I'm being demoted?
Are there any rules for identifying what spell an opponent is casting?
Why is gun control associated with the socially liberal Democratic party?
Threading data on TimeSeries
Can an escape pod land on Earth from orbit and not be immediately detected?
Digital signature that is only verifiable by one specific person
Does PC weight have a mechanical effect?
The title "Mord mit Aussicht" explained
Does anyone recognize these rockets, and their location?
How to avoid offending original culture when making conculture inspired from original
Why does MAGMA claim that the automorphism group of an elliptic curve is order 24 when it is order 12?
Why can't we feel the Earth's revolution?
Does the use of English words weaken diceware passphrases
Fastest path on a snakes and ladders board
Someone who is granted access to information but not expected to read it
How would Japanese people react to someone refusing to say “itadakimasu” for religious reasons?
Interview was just a one hour panel. Got an offer the next day; do I accept or is this a red flag?
Can an open source licence be revoked if it violates employer's IP?
For Saintsbury, which English novelists constituted the "great quartet of the mid-eighteenth century"?
How can I detect if I'm in a subshell?
Why not make one big CPU core?
How can I improve readability and length of a method with many if statements?
How can I concatenate two arrays in Java?How can I generate an MD5 hash?How can I pad an integer with zeros on the left?How can I initialise a static Map?How can I create an executable JAR with dependencies using Maven?How can I convert a stack trace to a string?How to make mock to void methods with MockitoHow to convert byte size into human readable format in java?How to reference a method in javadoc?Java sorting based on object one property with some condition
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty height:90px;width:728px;box-sizing:border-box;
I have a method with 195 if's. Here is a shorter version:
private BigDecimal calculateTax(String country, BigDecimal amount) throws Exception
if(country.equals("POLAND"))
return new BigDecimal(0.23).multiply(amount);
else if(country.equals("AUSTRIA"))
return new BigDecimal(0.20).multiply(amount);
else if(country.equals("CYPRUS"))
return new BigDecimal(0.19).multiply(amount);
else
throw new Exception("Country not supported");
I can change if's to switches:
private BigDecimal calculateTax(String country, BigDecimal amount) throws Exception
switch (country)
case "POLAND":
return new BigDecimal(0.23).multiply(amount);
case "AUSTRIA":
return new BigDecimal(0.20).multiply(amount);
case "CYPRUS":
return new BigDecimal(0.19).multiply(amount);
default:
throw new Exception("Country not supported");
but 195 cases is still so long. How could I improve readability and length of that method? What pattern would be the best in this case?
java design-patterns
|
show 1 more comment
I have a method with 195 if's. Here is a shorter version:
private BigDecimal calculateTax(String country, BigDecimal amount) throws Exception
if(country.equals("POLAND"))
return new BigDecimal(0.23).multiply(amount);
else if(country.equals("AUSTRIA"))
return new BigDecimal(0.20).multiply(amount);
else if(country.equals("CYPRUS"))
return new BigDecimal(0.19).multiply(amount);
else
throw new Exception("Country not supported");
I can change if's to switches:
private BigDecimal calculateTax(String country, BigDecimal amount) throws Exception
switch (country)
case "POLAND":
return new BigDecimal(0.23).multiply(amount);
case "AUSTRIA":
return new BigDecimal(0.20).multiply(amount);
case "CYPRUS":
return new BigDecimal(0.19).multiply(amount);
default:
throw new Exception("Country not supported");
but 195 cases is still so long. How could I improve readability and length of that method? What pattern would be the best in this case?
java design-patterns
15
Usejava.util.Map
... ?
– Oleksandr
9 hours ago
1
Yeah, a map or a dictionary is probably your best bet here.
– ncbvs
9 hours ago
Good point guys, but can I also put a design pattern here? Let's say that I am forced to
– Michu93
9 hours ago
You would probably be best off loading in a csv from disk, then storing it in a map.
– JClassic
9 hours ago
6
Warning: avoid using float point numbers with BigDecimal. BigDecimal(0.23) might not be the same as BigDecimal(23)/BigDecimal(100). The latter is the correct representation of 23%.
– gudok
8 hours ago
|
show 1 more comment
I have a method with 195 if's. Here is a shorter version:
private BigDecimal calculateTax(String country, BigDecimal amount) throws Exception
if(country.equals("POLAND"))
return new BigDecimal(0.23).multiply(amount);
else if(country.equals("AUSTRIA"))
return new BigDecimal(0.20).multiply(amount);
else if(country.equals("CYPRUS"))
return new BigDecimal(0.19).multiply(amount);
else
throw new Exception("Country not supported");
I can change if's to switches:
private BigDecimal calculateTax(String country, BigDecimal amount) throws Exception
switch (country)
case "POLAND":
return new BigDecimal(0.23).multiply(amount);
case "AUSTRIA":
return new BigDecimal(0.20).multiply(amount);
case "CYPRUS":
return new BigDecimal(0.19).multiply(amount);
default:
throw new Exception("Country not supported");
but 195 cases is still so long. How could I improve readability and length of that method? What pattern would be the best in this case?
java design-patterns
I have a method with 195 if's. Here is a shorter version:
private BigDecimal calculateTax(String country, BigDecimal amount) throws Exception
if(country.equals("POLAND"))
return new BigDecimal(0.23).multiply(amount);
else if(country.equals("AUSTRIA"))
return new BigDecimal(0.20).multiply(amount);
else if(country.equals("CYPRUS"))
return new BigDecimal(0.19).multiply(amount);
else
throw new Exception("Country not supported");
I can change if's to switches:
private BigDecimal calculateTax(String country, BigDecimal amount) throws Exception
switch (country)
case "POLAND":
return new BigDecimal(0.23).multiply(amount);
case "AUSTRIA":
return new BigDecimal(0.20).multiply(amount);
case "CYPRUS":
return new BigDecimal(0.19).multiply(amount);
default:
throw new Exception("Country not supported");
but 195 cases is still so long. How could I improve readability and length of that method? What pattern would be the best in this case?
java design-patterns
java design-patterns
edited 19 mins ago


Laurel
4,847102239
4,847102239
asked 9 hours ago


Michu93Michu93
1,05311533
1,05311533
15
Usejava.util.Map
... ?
– Oleksandr
9 hours ago
1
Yeah, a map or a dictionary is probably your best bet here.
– ncbvs
9 hours ago
Good point guys, but can I also put a design pattern here? Let's say that I am forced to
– Michu93
9 hours ago
You would probably be best off loading in a csv from disk, then storing it in a map.
– JClassic
9 hours ago
6
Warning: avoid using float point numbers with BigDecimal. BigDecimal(0.23) might not be the same as BigDecimal(23)/BigDecimal(100). The latter is the correct representation of 23%.
– gudok
8 hours ago
|
show 1 more comment
15
Usejava.util.Map
... ?
– Oleksandr
9 hours ago
1
Yeah, a map or a dictionary is probably your best bet here.
– ncbvs
9 hours ago
Good point guys, but can I also put a design pattern here? Let's say that I am forced to
– Michu93
9 hours ago
You would probably be best off loading in a csv from disk, then storing it in a map.
– JClassic
9 hours ago
6
Warning: avoid using float point numbers with BigDecimal. BigDecimal(0.23) might not be the same as BigDecimal(23)/BigDecimal(100). The latter is the correct representation of 23%.
– gudok
8 hours ago
15
15
Use
java.util.Map
... ?– Oleksandr
9 hours ago
Use
java.util.Map
... ?– Oleksandr
9 hours ago
1
1
Yeah, a map or a dictionary is probably your best bet here.
– ncbvs
9 hours ago
Yeah, a map or a dictionary is probably your best bet here.
– ncbvs
9 hours ago
Good point guys, but can I also put a design pattern here? Let's say that I am forced to
– Michu93
9 hours ago
Good point guys, but can I also put a design pattern here? Let's say that I am forced to
– Michu93
9 hours ago
You would probably be best off loading in a csv from disk, then storing it in a map.
– JClassic
9 hours ago
You would probably be best off loading in a csv from disk, then storing it in a map.
– JClassic
9 hours ago
6
6
Warning: avoid using float point numbers with BigDecimal. BigDecimal(0.23) might not be the same as BigDecimal(23)/BigDecimal(100). The latter is the correct representation of 23%.
– gudok
8 hours ago
Warning: avoid using float point numbers with BigDecimal. BigDecimal(0.23) might not be the same as BigDecimal(23)/BigDecimal(100). The latter is the correct representation of 23%.
– gudok
8 hours ago
|
show 1 more comment
4 Answers
4
active
oldest
votes
Create a Map<String,Double>
that maps country names to their corresponding tax rates:
Map<String,Double> taxRates = new HashMap<> ();
taxRates.put("POLAND",0.23);
...
Use that Map
as follows:
private BigDecimal calculateTax(String country, BigDecimal amount) throws Exception
if (taxRates.containsKey(country))
return new BigDecimal(taxRates.get(country)).multiply(amount);
else
throw new Exception("Country not supported");
1
This doesn't seem like a reasonable place to throw a checked exception. I know that OP's code does this, but I'd still rather not propagate it.
– Ben P.
9 hours ago
4
@BenP. I see no issue with throwing a checked exception if you receive an unexpected/not supported input. I'd probably use some custom exception and not the base Exception class though.
– Eran
9 hours ago
Or alternativelyOptional.ofNullable(taxRateMap.get(country)) .mapping(taxRate -> new BigDecimal(taxRate).multiply(amount); .orElseThrow( () -> new Exception("Country " + country + " not supported"));
– davidxxx
7 hours ago
AnIllegalArgumentException
should be fine, but a plainException
is a no-go...
– Marco13
6 hours ago
add a comment |
Put the data in an XML file or database, then use it to populate a dictionary. That way you can change the data easily, and separate the data from your application logic. Or, just execute a SQL query in your method instead.
add a comment |
If the values are constant and are not meant to be changed regularly (which I doubt). I'd introduce a static metamodel using Enum:
public enum CountryList
AUSTRIA(BigDecimal.valueOf(0.20)),
CYPRUS(BigDecimal.valueOf(0.19)),
POLAND(BigDecimal.valueOf(0.23));
private final BigDecimal countryTax;
CountryList(BigDecimal countryTax)
this.countryTax = countryTax;
public BigDecimal getCountryTax()
return countryTax;
public static BigDecimal countryTaxOf(String countryName)
CountryList country = Arrays.stream(CountryList.values())
.filter(c -> c.name().equalsIgnoreCase(countryName))
.findAny()
.orElseThrow(() -> new IllegalArgumentException("Country is not found in the dictionary: " + countryName));
return country.getCountryTax();
Then
private BigDecimal calculateTax(String country, BigDecimal amount) throws Exception
return CountryList.countryTaxOf(country).multiply(amount);
It's readable, compile time safe, easily extendable with additional metadata per country and less boilerplate.
Why the stream?
– immibis
47 mins ago
add a comment |
You may consider creating two arrays. It is easy to implement. For instance, in this case you may use :
String[] countries = "Poland", "India", "Mexico",....;
And another array for the respective BigDecimalvalue.
BigDecimal[] taxrates = 0.23,0.5,0.75;
Notice that since both the arrays are parallel, the value of index for both the arrays would be same for whichever is selected. For instance, index = 1 would always point to India and 0.5.
In case you need to remove or add an element from the array, you can use a temporary array and copy the values. Then create a new array and copy the required values. You can also edit values using index like using SQL id. In case of dynamic and drastic changes I suggest using ArrayList where we can easily access get() and indexOf("") or remove("") methods.
There maybe better approaches but I personally find this easy to implement.
P.S. - Hashmap may also be used to solve the problem.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f56586432%2fhow-can-i-improve-readability-and-length-of-a-method-with-many-if-statements%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
Create a Map<String,Double>
that maps country names to their corresponding tax rates:
Map<String,Double> taxRates = new HashMap<> ();
taxRates.put("POLAND",0.23);
...
Use that Map
as follows:
private BigDecimal calculateTax(String country, BigDecimal amount) throws Exception
if (taxRates.containsKey(country))
return new BigDecimal(taxRates.get(country)).multiply(amount);
else
throw new Exception("Country not supported");
1
This doesn't seem like a reasonable place to throw a checked exception. I know that OP's code does this, but I'd still rather not propagate it.
– Ben P.
9 hours ago
4
@BenP. I see no issue with throwing a checked exception if you receive an unexpected/not supported input. I'd probably use some custom exception and not the base Exception class though.
– Eran
9 hours ago
Or alternativelyOptional.ofNullable(taxRateMap.get(country)) .mapping(taxRate -> new BigDecimal(taxRate).multiply(amount); .orElseThrow( () -> new Exception("Country " + country + " not supported"));
– davidxxx
7 hours ago
AnIllegalArgumentException
should be fine, but a plainException
is a no-go...
– Marco13
6 hours ago
add a comment |
Create a Map<String,Double>
that maps country names to their corresponding tax rates:
Map<String,Double> taxRates = new HashMap<> ();
taxRates.put("POLAND",0.23);
...
Use that Map
as follows:
private BigDecimal calculateTax(String country, BigDecimal amount) throws Exception
if (taxRates.containsKey(country))
return new BigDecimal(taxRates.get(country)).multiply(amount);
else
throw new Exception("Country not supported");
1
This doesn't seem like a reasonable place to throw a checked exception. I know that OP's code does this, but I'd still rather not propagate it.
– Ben P.
9 hours ago
4
@BenP. I see no issue with throwing a checked exception if you receive an unexpected/not supported input. I'd probably use some custom exception and not the base Exception class though.
– Eran
9 hours ago
Or alternativelyOptional.ofNullable(taxRateMap.get(country)) .mapping(taxRate -> new BigDecimal(taxRate).multiply(amount); .orElseThrow( () -> new Exception("Country " + country + " not supported"));
– davidxxx
7 hours ago
AnIllegalArgumentException
should be fine, but a plainException
is a no-go...
– Marco13
6 hours ago
add a comment |
Create a Map<String,Double>
that maps country names to their corresponding tax rates:
Map<String,Double> taxRates = new HashMap<> ();
taxRates.put("POLAND",0.23);
...
Use that Map
as follows:
private BigDecimal calculateTax(String country, BigDecimal amount) throws Exception
if (taxRates.containsKey(country))
return new BigDecimal(taxRates.get(country)).multiply(amount);
else
throw new Exception("Country not supported");
Create a Map<String,Double>
that maps country names to their corresponding tax rates:
Map<String,Double> taxRates = new HashMap<> ();
taxRates.put("POLAND",0.23);
...
Use that Map
as follows:
private BigDecimal calculateTax(String country, BigDecimal amount) throws Exception
if (taxRates.containsKey(country))
return new BigDecimal(taxRates.get(country)).multiply(amount);
else
throw new Exception("Country not supported");
edited 9 hours ago
answered 9 hours ago


EranEran
299k37502581
299k37502581
1
This doesn't seem like a reasonable place to throw a checked exception. I know that OP's code does this, but I'd still rather not propagate it.
– Ben P.
9 hours ago
4
@BenP. I see no issue with throwing a checked exception if you receive an unexpected/not supported input. I'd probably use some custom exception and not the base Exception class though.
– Eran
9 hours ago
Or alternativelyOptional.ofNullable(taxRateMap.get(country)) .mapping(taxRate -> new BigDecimal(taxRate).multiply(amount); .orElseThrow( () -> new Exception("Country " + country + " not supported"));
– davidxxx
7 hours ago
AnIllegalArgumentException
should be fine, but a plainException
is a no-go...
– Marco13
6 hours ago
add a comment |
1
This doesn't seem like a reasonable place to throw a checked exception. I know that OP's code does this, but I'd still rather not propagate it.
– Ben P.
9 hours ago
4
@BenP. I see no issue with throwing a checked exception if you receive an unexpected/not supported input. I'd probably use some custom exception and not the base Exception class though.
– Eran
9 hours ago
Or alternativelyOptional.ofNullable(taxRateMap.get(country)) .mapping(taxRate -> new BigDecimal(taxRate).multiply(amount); .orElseThrow( () -> new Exception("Country " + country + " not supported"));
– davidxxx
7 hours ago
AnIllegalArgumentException
should be fine, but a plainException
is a no-go...
– Marco13
6 hours ago
1
1
This doesn't seem like a reasonable place to throw a checked exception. I know that OP's code does this, but I'd still rather not propagate it.
– Ben P.
9 hours ago
This doesn't seem like a reasonable place to throw a checked exception. I know that OP's code does this, but I'd still rather not propagate it.
– Ben P.
9 hours ago
4
4
@BenP. I see no issue with throwing a checked exception if you receive an unexpected/not supported input. I'd probably use some custom exception and not the base Exception class though.
– Eran
9 hours ago
@BenP. I see no issue with throwing a checked exception if you receive an unexpected/not supported input. I'd probably use some custom exception and not the base Exception class though.
– Eran
9 hours ago
Or alternatively
Optional.ofNullable(taxRateMap.get(country)) .mapping(taxRate -> new BigDecimal(taxRate).multiply(amount); .orElseThrow( () -> new Exception("Country " + country + " not supported"));
– davidxxx
7 hours ago
Or alternatively
Optional.ofNullable(taxRateMap.get(country)) .mapping(taxRate -> new BigDecimal(taxRate).multiply(amount); .orElseThrow( () -> new Exception("Country " + country + " not supported"));
– davidxxx
7 hours ago
An
IllegalArgumentException
should be fine, but a plain Exception
is a no-go...– Marco13
6 hours ago
An
IllegalArgumentException
should be fine, but a plain Exception
is a no-go...– Marco13
6 hours ago
add a comment |
Put the data in an XML file or database, then use it to populate a dictionary. That way you can change the data easily, and separate the data from your application logic. Or, just execute a SQL query in your method instead.
add a comment |
Put the data in an XML file or database, then use it to populate a dictionary. That way you can change the data easily, and separate the data from your application logic. Or, just execute a SQL query in your method instead.
add a comment |
Put the data in an XML file or database, then use it to populate a dictionary. That way you can change the data easily, and separate the data from your application logic. Or, just execute a SQL query in your method instead.
Put the data in an XML file or database, then use it to populate a dictionary. That way you can change the data easily, and separate the data from your application logic. Or, just execute a SQL query in your method instead.
answered 9 hours ago


EJoshuaSEJoshuaS
7,622103151
7,622103151
add a comment |
add a comment |
If the values are constant and are not meant to be changed regularly (which I doubt). I'd introduce a static metamodel using Enum:
public enum CountryList
AUSTRIA(BigDecimal.valueOf(0.20)),
CYPRUS(BigDecimal.valueOf(0.19)),
POLAND(BigDecimal.valueOf(0.23));
private final BigDecimal countryTax;
CountryList(BigDecimal countryTax)
this.countryTax = countryTax;
public BigDecimal getCountryTax()
return countryTax;
public static BigDecimal countryTaxOf(String countryName)
CountryList country = Arrays.stream(CountryList.values())
.filter(c -> c.name().equalsIgnoreCase(countryName))
.findAny()
.orElseThrow(() -> new IllegalArgumentException("Country is not found in the dictionary: " + countryName));
return country.getCountryTax();
Then
private BigDecimal calculateTax(String country, BigDecimal amount) throws Exception
return CountryList.countryTaxOf(country).multiply(amount);
It's readable, compile time safe, easily extendable with additional metadata per country and less boilerplate.
Why the stream?
– immibis
47 mins ago
add a comment |
If the values are constant and are not meant to be changed regularly (which I doubt). I'd introduce a static metamodel using Enum:
public enum CountryList
AUSTRIA(BigDecimal.valueOf(0.20)),
CYPRUS(BigDecimal.valueOf(0.19)),
POLAND(BigDecimal.valueOf(0.23));
private final BigDecimal countryTax;
CountryList(BigDecimal countryTax)
this.countryTax = countryTax;
public BigDecimal getCountryTax()
return countryTax;
public static BigDecimal countryTaxOf(String countryName)
CountryList country = Arrays.stream(CountryList.values())
.filter(c -> c.name().equalsIgnoreCase(countryName))
.findAny()
.orElseThrow(() -> new IllegalArgumentException("Country is not found in the dictionary: " + countryName));
return country.getCountryTax();
Then
private BigDecimal calculateTax(String country, BigDecimal amount) throws Exception
return CountryList.countryTaxOf(country).multiply(amount);
It's readable, compile time safe, easily extendable with additional metadata per country and less boilerplate.
Why the stream?
– immibis
47 mins ago
add a comment |
If the values are constant and are not meant to be changed regularly (which I doubt). I'd introduce a static metamodel using Enum:
public enum CountryList
AUSTRIA(BigDecimal.valueOf(0.20)),
CYPRUS(BigDecimal.valueOf(0.19)),
POLAND(BigDecimal.valueOf(0.23));
private final BigDecimal countryTax;
CountryList(BigDecimal countryTax)
this.countryTax = countryTax;
public BigDecimal getCountryTax()
return countryTax;
public static BigDecimal countryTaxOf(String countryName)
CountryList country = Arrays.stream(CountryList.values())
.filter(c -> c.name().equalsIgnoreCase(countryName))
.findAny()
.orElseThrow(() -> new IllegalArgumentException("Country is not found in the dictionary: " + countryName));
return country.getCountryTax();
Then
private BigDecimal calculateTax(String country, BigDecimal amount) throws Exception
return CountryList.countryTaxOf(country).multiply(amount);
It's readable, compile time safe, easily extendable with additional metadata per country and less boilerplate.
If the values are constant and are not meant to be changed regularly (which I doubt). I'd introduce a static metamodel using Enum:
public enum CountryList
AUSTRIA(BigDecimal.valueOf(0.20)),
CYPRUS(BigDecimal.valueOf(0.19)),
POLAND(BigDecimal.valueOf(0.23));
private final BigDecimal countryTax;
CountryList(BigDecimal countryTax)
this.countryTax = countryTax;
public BigDecimal getCountryTax()
return countryTax;
public static BigDecimal countryTaxOf(String countryName)
CountryList country = Arrays.stream(CountryList.values())
.filter(c -> c.name().equalsIgnoreCase(countryName))
.findAny()
.orElseThrow(() -> new IllegalArgumentException("Country is not found in the dictionary: " + countryName));
return country.getCountryTax();
Then
private BigDecimal calculateTax(String country, BigDecimal amount) throws Exception
return CountryList.countryTaxOf(country).multiply(amount);
It's readable, compile time safe, easily extendable with additional metadata per country and less boilerplate.
answered 7 hours ago
Mikhail KholodkovMikhail Kholodkov
6,18563154
6,18563154
Why the stream?
– immibis
47 mins ago
add a comment |
Why the stream?
– immibis
47 mins ago
Why the stream?
– immibis
47 mins ago
Why the stream?
– immibis
47 mins ago
add a comment |
You may consider creating two arrays. It is easy to implement. For instance, in this case you may use :
String[] countries = "Poland", "India", "Mexico",....;
And another array for the respective BigDecimalvalue.
BigDecimal[] taxrates = 0.23,0.5,0.75;
Notice that since both the arrays are parallel, the value of index for both the arrays would be same for whichever is selected. For instance, index = 1 would always point to India and 0.5.
In case you need to remove or add an element from the array, you can use a temporary array and copy the values. Then create a new array and copy the required values. You can also edit values using index like using SQL id. In case of dynamic and drastic changes I suggest using ArrayList where we can easily access get() and indexOf("") or remove("") methods.
There maybe better approaches but I personally find this easy to implement.
P.S. - Hashmap may also be used to solve the problem.
add a comment |
You may consider creating two arrays. It is easy to implement. For instance, in this case you may use :
String[] countries = "Poland", "India", "Mexico",....;
And another array for the respective BigDecimalvalue.
BigDecimal[] taxrates = 0.23,0.5,0.75;
Notice that since both the arrays are parallel, the value of index for both the arrays would be same for whichever is selected. For instance, index = 1 would always point to India and 0.5.
In case you need to remove or add an element from the array, you can use a temporary array and copy the values. Then create a new array and copy the required values. You can also edit values using index like using SQL id. In case of dynamic and drastic changes I suggest using ArrayList where we can easily access get() and indexOf("") or remove("") methods.
There maybe better approaches but I personally find this easy to implement.
P.S. - Hashmap may also be used to solve the problem.
add a comment |
You may consider creating two arrays. It is easy to implement. For instance, in this case you may use :
String[] countries = "Poland", "India", "Mexico",....;
And another array for the respective BigDecimalvalue.
BigDecimal[] taxrates = 0.23,0.5,0.75;
Notice that since both the arrays are parallel, the value of index for both the arrays would be same for whichever is selected. For instance, index = 1 would always point to India and 0.5.
In case you need to remove or add an element from the array, you can use a temporary array and copy the values. Then create a new array and copy the required values. You can also edit values using index like using SQL id. In case of dynamic and drastic changes I suggest using ArrayList where we can easily access get() and indexOf("") or remove("") methods.
There maybe better approaches but I personally find this easy to implement.
P.S. - Hashmap may also be used to solve the problem.
You may consider creating two arrays. It is easy to implement. For instance, in this case you may use :
String[] countries = "Poland", "India", "Mexico",....;
And another array for the respective BigDecimalvalue.
BigDecimal[] taxrates = 0.23,0.5,0.75;
Notice that since both the arrays are parallel, the value of index for both the arrays would be same for whichever is selected. For instance, index = 1 would always point to India and 0.5.
In case you need to remove or add an element from the array, you can use a temporary array and copy the values. Then create a new array and copy the required values. You can also edit values using index like using SQL id. In case of dynamic and drastic changes I suggest using ArrayList where we can easily access get() and indexOf("") or remove("") methods.
There maybe better approaches but I personally find this easy to implement.
P.S. - Hashmap may also be used to solve the problem.
answered 8 hours ago
HeisenbergHeisenberg
133
133
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f56586432%2fhow-can-i-improve-readability-and-length-of-a-method-with-many-if-statements%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
WS9xJo5L uHQPiO1XiE76fGhBxmgybg,Be7v1bOcGiDAIwu,BUb6vH S3F,uW53BxisCwFlY55PG yh 3,M,HtB4GXpa7A0pcOub u8WCGaTV
15
Use
java.util.Map
... ?– Oleksandr
9 hours ago
1
Yeah, a map or a dictionary is probably your best bet here.
– ncbvs
9 hours ago
Good point guys, but can I also put a design pattern here? Let's say that I am forced to
– Michu93
9 hours ago
You would probably be best off loading in a csv from disk, then storing it in a map.
– JClassic
9 hours ago
6
Warning: avoid using float point numbers with BigDecimal. BigDecimal(0.23) might not be the same as BigDecimal(23)/BigDecimal(100). The latter is the correct representation of 23%.
– gudok
8 hours ago