Why does it output Integers instead of letters?In Java, is the result of the addition of two chars an int or a char?String builder vs string concatenationWhat is a serialVersionUID and why should I use it?How do I generate random integers within a specific range in Java?Python join: why is it string.join(list) instead of list.join(string)?How do I make the first letter of a string uppercase in JavaScript?Does Python have a string 'contains' substring method?Why is subtracting these two times (in 1927) giving a strange result?Why don't Java's +=, -=, *=, /= compound assignment operators require casting?Why is char[] preferred over String for passwords?Why is processing a sorted array faster than processing an unsorted array?Why does this code using random strings print “hello world”?
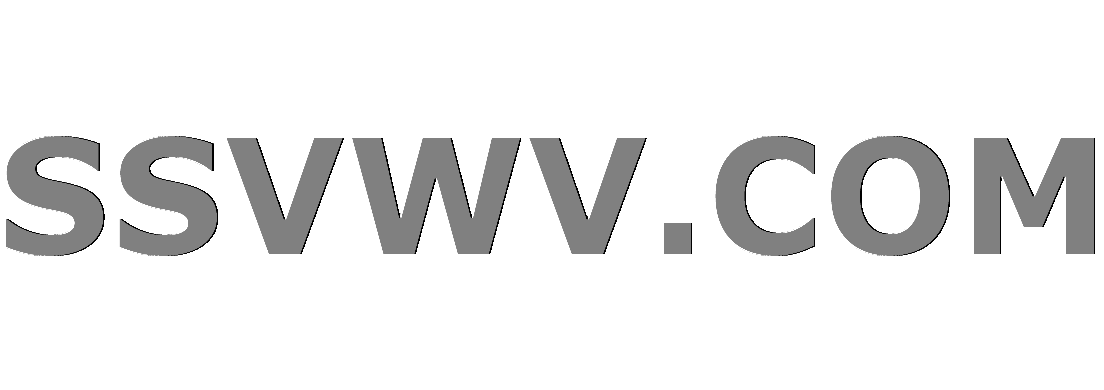
Multi tool use
definition of "percentile"
RPI3B+: What are the four components below the HDMI connector called?
Is "I do not want you to go nowhere" a case of "DOUBLE-NEGATIVES" as claimed by Grammarly?
How do you glue a text to a point?
Misspelling my name on my mathematical publications
How would vampires avoid contracting diseases?
Why does the U.S. tolerate foreign influence from Saudi Arabia and Israel on its domestic policies while not tolerating that from China or Russia?
Should disabled buttons give feedback when clicked?
How to convert a file with several spaces into a tab-delimited file?
Integer Lists of Noah
Confirming the Identity of a (Friendly) Reviewer After the Reviews
How to memorize multiple pieces?
What is a solution?
What is this triple-transistor arrangement called?
Is the genetic term "polycistronic" still used in modern biology?
Constructive proof of existence of free algebras for infinitary equational theories
Why are all my yellow 2V/20mA LEDs burning out with 330k Ohm resistor?
Print the last, middle and first character of your code
Is Trump personally blocking people on Twitter?
Is a request to book a business flight ticket for a graduate student an unreasonable one?
Using Newton's shell theorem to accelerate a spaceship
How to loop for 3 times in bash script when docker push fails?
How can I effectively communicate to recruiters that a phone call is not possible?
For a hashing function like MD5, how similar can two plaintext strings be and still generate the same hash?
Why does it output Integers instead of letters?
In Java, is the result of the addition of two chars an int or a char?String builder vs string concatenationWhat is a serialVersionUID and why should I use it?How do I generate random integers within a specific range in Java?Python join: why is it string.join(list) instead of list.join(string)?How do I make the first letter of a string uppercase in JavaScript?Does Python have a string 'contains' substring method?Why is subtracting these two times (in 1927) giving a strange result?Why don't Java's +=, -=, *=, /= compound assignment operators require casting?Why is char[] preferred over String for passwords?Why is processing a sorted array faster than processing an unsorted array?Why does this code using random strings print “hello world”?
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty margin-bottom:0;
So this is the task: Given a string, return a string where for every char in the original, there are two chars.
And I don't understand why its output are numbers instead of letters, I tried doesn't work?
public String doubleChar(String str)
String s = "";
for(int i=0; i<str.length(); i++)
s += str.charAt(i) + str.charAt(i);
return s;
Expected :
doubleChar("The") → "TThhee"
doubleChar("AAbb") → "AAAAbbbb"
Output:
doubleChar("The") → "168208202"
doubleChar("AAbb") → "130130196196"
java string
add a comment |
So this is the task: Given a string, return a string where for every char in the original, there are two chars.
And I don't understand why its output are numbers instead of letters, I tried doesn't work?
public String doubleChar(String str)
String s = "";
for(int i=0; i<str.length(); i++)
s += str.charAt(i) + str.charAt(i);
return s;
Expected :
doubleChar("The") → "TThhee"
doubleChar("AAbb") → "AAAAbbbb"
Output:
doubleChar("The") → "168208202"
doubleChar("AAbb") → "130130196196"
java string
5
Adding twochar
s doesn't give you a string with those two characters, but rather an int with numerical value equal to the sum of the two characters' numerical values.
– Andrey Akhmetov
8 hours ago
add a comment |
So this is the task: Given a string, return a string where for every char in the original, there are two chars.
And I don't understand why its output are numbers instead of letters, I tried doesn't work?
public String doubleChar(String str)
String s = "";
for(int i=0; i<str.length(); i++)
s += str.charAt(i) + str.charAt(i);
return s;
Expected :
doubleChar("The") → "TThhee"
doubleChar("AAbb") → "AAAAbbbb"
Output:
doubleChar("The") → "168208202"
doubleChar("AAbb") → "130130196196"
java string
So this is the task: Given a string, return a string where for every char in the original, there are two chars.
And I don't understand why its output are numbers instead of letters, I tried doesn't work?
public String doubleChar(String str)
String s = "";
for(int i=0; i<str.length(); i++)
s += str.charAt(i) + str.charAt(i);
return s;
Expected :
doubleChar("The") → "TThhee"
doubleChar("AAbb") → "AAAAbbbb"
Output:
doubleChar("The") → "168208202"
doubleChar("AAbb") → "130130196196"
java string
java string
edited 8 hours ago
stevie lol
asked 8 hours ago
stevie lolstevie lol
413 bronze badges
413 bronze badges
5
Adding twochar
s doesn't give you a string with those two characters, but rather an int with numerical value equal to the sum of the two characters' numerical values.
– Andrey Akhmetov
8 hours ago
add a comment |
5
Adding twochar
s doesn't give you a string with those two characters, but rather an int with numerical value equal to the sum of the two characters' numerical values.
– Andrey Akhmetov
8 hours ago
5
5
Adding two
char
s doesn't give you a string with those two characters, but rather an int with numerical value equal to the sum of the two characters' numerical values.– Andrey Akhmetov
8 hours ago
Adding two
char
s doesn't give you a string with those two characters, but rather an int with numerical value equal to the sum of the two characters' numerical values.– Andrey Akhmetov
8 hours ago
add a comment |
5 Answers
5
active
oldest
votes
In Java the char
primitive type is basically just a numeric value that maps to a character, so if you add two char
values together they produce a number and not another char
(and not a String
) so you end up with an int
as you're seeing.
To fix this you can use the Character.toString(char)
method like this:
s += Character.toString(str.charAt(i)) + Character.toString(str.charAt(i))
But this is all fairly inefficient because you're doing this in a loop and so string concatenation is producing a lot of String
objects needlessly. More efficient is to use a StringBuilder
and its append(char)
method like this:
StringBuilder sb = new StringBuilder(str.length() * 2);
for (int i = 0; i < str.length(); ++i)
char c = str.charAt(i);
sb.append(c).append(c);
return sb.toString();
1
This should be correct answer. Creating new String objects each loop iteration is not good practice. Using a StringBuilder is more efficient. Look here for exaplanation stackoverflow.com/a/18453485/8685250
– Hayes Roach
7 hours ago
add a comment |
You are adding numeric values of chars first before concatenating the result (now integer) to the string. Try debugging with print statements:
public static String doubleChar(String str)
String s = "";
for (int i = 0; i < str.length(); i++)
System.out.println(str.charAt(i));
System.out.println(str.charAt(i) + str.charAt(i));
s += str.charAt(i) + str.charAt(i);
return s;
The more efficient way of doing what you want is:
public static String doubleChar(String str)
StringBuilder sb = new StringBuilder(str.length() * 2);
for (int i = 0; i < str.length(); i++)
char c = str.charAt(i);
sb.append(c).append(c);
return sb.toString();
2
I agree he should be usingStringBuilder
since he is concatenating aString
inside of a loop, but it may be helpful to explain briefly why it is better.
– Nexevis
8 hours ago
add a comment |
Why does it output integers?
The + operator is overloaded in Java to perform String concatenation only for String
s, not char
s.
From the Java Spec:
If the type of either operand of a + operator is String, then the
operation is string concatenation.
Otherwise, the type of each of the operands of the + operator must be
a type that is convertible (§5.1.8) to a primitive numeric type, or a
compile-time error occurs.
In your case, char is converted to its primitive value (int), then added.
Instead, use StringBuilder.append(char)
to concatenate them into a String
.
If performance is not a concern, you could even do:
char c = 'A';
String s = "" + c + c;
and s += "" + c + c;
That will force the + String
concatenation operator because it starts with a String
(""). The Java Spec above explains with examples:
The + operator is syntactically left-associative, no matter whether it
is determined by type analysis to represent string concatenation or
numeric addition. In some cases care is required to get the desired
result. For example [...]
1 + 2 + " fiddlers"
is"3 fiddlers"
but the result of:
"fiddlers " + 1 + 2
is"fiddlers 12"
add a comment |
You are adding the value of two characters together. Change the String
concatenation from:
s += str.charAt(i) + str.charAt(i);
To:
s += str.charAt(i) + "" + str.charAt(i);
Which will ensure the characters convert to a String
.
Note: This is a quick fix, and you should use StringBuilder
when String
concatenating inside of a loop. See the other answers for how this is done.
add a comment |
String.charAt()
returns a char
(https://docs.oracle.com/javase/7/docs/api/java/lang/String.html#charAt(int)) so you're dealing with a single character. With onechar
, you can perform operations on it like this (which will print "65" which is ASCII value for the 'A' character):
System.out.println('A' + 0);
you can print the ASCII value for the next character ("B") by adding 1 to 'A'
, like this:
System.out.println('A' + 1);
To make your code work – so that it doubles each character – there are number of options. You could append each character one at a time:
s += str.charAt(i);
s += str.charAt(i);
or various ways of casting the operation to a string:
s += "" + str.charAt(i) + str.charAt(i);
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f56977717%2fwhy-does-it-output-integers-instead-of-letters%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
5 Answers
5
active
oldest
votes
5 Answers
5
active
oldest
votes
active
oldest
votes
active
oldest
votes
In Java the char
primitive type is basically just a numeric value that maps to a character, so if you add two char
values together they produce a number and not another char
(and not a String
) so you end up with an int
as you're seeing.
To fix this you can use the Character.toString(char)
method like this:
s += Character.toString(str.charAt(i)) + Character.toString(str.charAt(i))
But this is all fairly inefficient because you're doing this in a loop and so string concatenation is producing a lot of String
objects needlessly. More efficient is to use a StringBuilder
and its append(char)
method like this:
StringBuilder sb = new StringBuilder(str.length() * 2);
for (int i = 0; i < str.length(); ++i)
char c = str.charAt(i);
sb.append(c).append(c);
return sb.toString();
1
This should be correct answer. Creating new String objects each loop iteration is not good practice. Using a StringBuilder is more efficient. Look here for exaplanation stackoverflow.com/a/18453485/8685250
– Hayes Roach
7 hours ago
add a comment |
In Java the char
primitive type is basically just a numeric value that maps to a character, so if you add two char
values together they produce a number and not another char
(and not a String
) so you end up with an int
as you're seeing.
To fix this you can use the Character.toString(char)
method like this:
s += Character.toString(str.charAt(i)) + Character.toString(str.charAt(i))
But this is all fairly inefficient because you're doing this in a loop and so string concatenation is producing a lot of String
objects needlessly. More efficient is to use a StringBuilder
and its append(char)
method like this:
StringBuilder sb = new StringBuilder(str.length() * 2);
for (int i = 0; i < str.length(); ++i)
char c = str.charAt(i);
sb.append(c).append(c);
return sb.toString();
1
This should be correct answer. Creating new String objects each loop iteration is not good practice. Using a StringBuilder is more efficient. Look here for exaplanation stackoverflow.com/a/18453485/8685250
– Hayes Roach
7 hours ago
add a comment |
In Java the char
primitive type is basically just a numeric value that maps to a character, so if you add two char
values together they produce a number and not another char
(and not a String
) so you end up with an int
as you're seeing.
To fix this you can use the Character.toString(char)
method like this:
s += Character.toString(str.charAt(i)) + Character.toString(str.charAt(i))
But this is all fairly inefficient because you're doing this in a loop and so string concatenation is producing a lot of String
objects needlessly. More efficient is to use a StringBuilder
and its append(char)
method like this:
StringBuilder sb = new StringBuilder(str.length() * 2);
for (int i = 0; i < str.length(); ++i)
char c = str.charAt(i);
sb.append(c).append(c);
return sb.toString();
In Java the char
primitive type is basically just a numeric value that maps to a character, so if you add two char
values together they produce a number and not another char
(and not a String
) so you end up with an int
as you're seeing.
To fix this you can use the Character.toString(char)
method like this:
s += Character.toString(str.charAt(i)) + Character.toString(str.charAt(i))
But this is all fairly inefficient because you're doing this in a loop and so string concatenation is producing a lot of String
objects needlessly. More efficient is to use a StringBuilder
and its append(char)
method like this:
StringBuilder sb = new StringBuilder(str.length() * 2);
for (int i = 0; i < str.length(); ++i)
char c = str.charAt(i);
sb.append(c).append(c);
return sb.toString();
answered 8 hours ago


BobulousBobulous
10.2k4 gold badges30 silver badges53 bronze badges
10.2k4 gold badges30 silver badges53 bronze badges
1
This should be correct answer. Creating new String objects each loop iteration is not good practice. Using a StringBuilder is more efficient. Look here for exaplanation stackoverflow.com/a/18453485/8685250
– Hayes Roach
7 hours ago
add a comment |
1
This should be correct answer. Creating new String objects each loop iteration is not good practice. Using a StringBuilder is more efficient. Look here for exaplanation stackoverflow.com/a/18453485/8685250
– Hayes Roach
7 hours ago
1
1
This should be correct answer. Creating new String objects each loop iteration is not good practice. Using a StringBuilder is more efficient. Look here for exaplanation stackoverflow.com/a/18453485/8685250
– Hayes Roach
7 hours ago
This should be correct answer. Creating new String objects each loop iteration is not good practice. Using a StringBuilder is more efficient. Look here for exaplanation stackoverflow.com/a/18453485/8685250
– Hayes Roach
7 hours ago
add a comment |
You are adding numeric values of chars first before concatenating the result (now integer) to the string. Try debugging with print statements:
public static String doubleChar(String str)
String s = "";
for (int i = 0; i < str.length(); i++)
System.out.println(str.charAt(i));
System.out.println(str.charAt(i) + str.charAt(i));
s += str.charAt(i) + str.charAt(i);
return s;
The more efficient way of doing what you want is:
public static String doubleChar(String str)
StringBuilder sb = new StringBuilder(str.length() * 2);
for (int i = 0; i < str.length(); i++)
char c = str.charAt(i);
sb.append(c).append(c);
return sb.toString();
2
I agree he should be usingStringBuilder
since he is concatenating aString
inside of a loop, but it may be helpful to explain briefly why it is better.
– Nexevis
8 hours ago
add a comment |
You are adding numeric values of chars first before concatenating the result (now integer) to the string. Try debugging with print statements:
public static String doubleChar(String str)
String s = "";
for (int i = 0; i < str.length(); i++)
System.out.println(str.charAt(i));
System.out.println(str.charAt(i) + str.charAt(i));
s += str.charAt(i) + str.charAt(i);
return s;
The more efficient way of doing what you want is:
public static String doubleChar(String str)
StringBuilder sb = new StringBuilder(str.length() * 2);
for (int i = 0; i < str.length(); i++)
char c = str.charAt(i);
sb.append(c).append(c);
return sb.toString();
2
I agree he should be usingStringBuilder
since he is concatenating aString
inside of a loop, but it may be helpful to explain briefly why it is better.
– Nexevis
8 hours ago
add a comment |
You are adding numeric values of chars first before concatenating the result (now integer) to the string. Try debugging with print statements:
public static String doubleChar(String str)
String s = "";
for (int i = 0; i < str.length(); i++)
System.out.println(str.charAt(i));
System.out.println(str.charAt(i) + str.charAt(i));
s += str.charAt(i) + str.charAt(i);
return s;
The more efficient way of doing what you want is:
public static String doubleChar(String str)
StringBuilder sb = new StringBuilder(str.length() * 2);
for (int i = 0; i < str.length(); i++)
char c = str.charAt(i);
sb.append(c).append(c);
return sb.toString();
You are adding numeric values of chars first before concatenating the result (now integer) to the string. Try debugging with print statements:
public static String doubleChar(String str)
String s = "";
for (int i = 0; i < str.length(); i++)
System.out.println(str.charAt(i));
System.out.println(str.charAt(i) + str.charAt(i));
s += str.charAt(i) + str.charAt(i);
return s;
The more efficient way of doing what you want is:
public static String doubleChar(String str)
StringBuilder sb = new StringBuilder(str.length() * 2);
for (int i = 0; i < str.length(); i++)
char c = str.charAt(i);
sb.append(c).append(c);
return sb.toString();
answered 8 hours ago
LeffeBruneLeffeBrune
2,5221 gold badge18 silver badges30 bronze badges
2,5221 gold badge18 silver badges30 bronze badges
2
I agree he should be usingStringBuilder
since he is concatenating aString
inside of a loop, but it may be helpful to explain briefly why it is better.
– Nexevis
8 hours ago
add a comment |
2
I agree he should be usingStringBuilder
since he is concatenating aString
inside of a loop, but it may be helpful to explain briefly why it is better.
– Nexevis
8 hours ago
2
2
I agree he should be using
StringBuilder
since he is concatenating a String
inside of a loop, but it may be helpful to explain briefly why it is better.– Nexevis
8 hours ago
I agree he should be using
StringBuilder
since he is concatenating a String
inside of a loop, but it may be helpful to explain briefly why it is better.– Nexevis
8 hours ago
add a comment |
Why does it output integers?
The + operator is overloaded in Java to perform String concatenation only for String
s, not char
s.
From the Java Spec:
If the type of either operand of a + operator is String, then the
operation is string concatenation.
Otherwise, the type of each of the operands of the + operator must be
a type that is convertible (§5.1.8) to a primitive numeric type, or a
compile-time error occurs.
In your case, char is converted to its primitive value (int), then added.
Instead, use StringBuilder.append(char)
to concatenate them into a String
.
If performance is not a concern, you could even do:
char c = 'A';
String s = "" + c + c;
and s += "" + c + c;
That will force the + String
concatenation operator because it starts with a String
(""). The Java Spec above explains with examples:
The + operator is syntactically left-associative, no matter whether it
is determined by type analysis to represent string concatenation or
numeric addition. In some cases care is required to get the desired
result. For example [...]
1 + 2 + " fiddlers"
is"3 fiddlers"
but the result of:
"fiddlers " + 1 + 2
is"fiddlers 12"
add a comment |
Why does it output integers?
The + operator is overloaded in Java to perform String concatenation only for String
s, not char
s.
From the Java Spec:
If the type of either operand of a + operator is String, then the
operation is string concatenation.
Otherwise, the type of each of the operands of the + operator must be
a type that is convertible (§5.1.8) to a primitive numeric type, or a
compile-time error occurs.
In your case, char is converted to its primitive value (int), then added.
Instead, use StringBuilder.append(char)
to concatenate them into a String
.
If performance is not a concern, you could even do:
char c = 'A';
String s = "" + c + c;
and s += "" + c + c;
That will force the + String
concatenation operator because it starts with a String
(""). The Java Spec above explains with examples:
The + operator is syntactically left-associative, no matter whether it
is determined by type analysis to represent string concatenation or
numeric addition. In some cases care is required to get the desired
result. For example [...]
1 + 2 + " fiddlers"
is"3 fiddlers"
but the result of:
"fiddlers " + 1 + 2
is"fiddlers 12"
add a comment |
Why does it output integers?
The + operator is overloaded in Java to perform String concatenation only for String
s, not char
s.
From the Java Spec:
If the type of either operand of a + operator is String, then the
operation is string concatenation.
Otherwise, the type of each of the operands of the + operator must be
a type that is convertible (§5.1.8) to a primitive numeric type, or a
compile-time error occurs.
In your case, char is converted to its primitive value (int), then added.
Instead, use StringBuilder.append(char)
to concatenate them into a String
.
If performance is not a concern, you could even do:
char c = 'A';
String s = "" + c + c;
and s += "" + c + c;
That will force the + String
concatenation operator because it starts with a String
(""). The Java Spec above explains with examples:
The + operator is syntactically left-associative, no matter whether it
is determined by type analysis to represent string concatenation or
numeric addition. In some cases care is required to get the desired
result. For example [...]
1 + 2 + " fiddlers"
is"3 fiddlers"
but the result of:
"fiddlers " + 1 + 2
is"fiddlers 12"
Why does it output integers?
The + operator is overloaded in Java to perform String concatenation only for String
s, not char
s.
From the Java Spec:
If the type of either operand of a + operator is String, then the
operation is string concatenation.
Otherwise, the type of each of the operands of the + operator must be
a type that is convertible (§5.1.8) to a primitive numeric type, or a
compile-time error occurs.
In your case, char is converted to its primitive value (int), then added.
Instead, use StringBuilder.append(char)
to concatenate them into a String
.
If performance is not a concern, you could even do:
char c = 'A';
String s = "" + c + c;
and s += "" + c + c;
That will force the + String
concatenation operator because it starts with a String
(""). The Java Spec above explains with examples:
The + operator is syntactically left-associative, no matter whether it
is determined by type analysis to represent string concatenation or
numeric addition. In some cases care is required to get the desired
result. For example [...]
1 + 2 + " fiddlers"
is"3 fiddlers"
but the result of:
"fiddlers " + 1 + 2
is"fiddlers 12"
edited 5 hours ago
answered 8 hours ago
EdwardEdward
414 bronze badges
414 bronze badges
add a comment |
add a comment |
You are adding the value of two characters together. Change the String
concatenation from:
s += str.charAt(i) + str.charAt(i);
To:
s += str.charAt(i) + "" + str.charAt(i);
Which will ensure the characters convert to a String
.
Note: This is a quick fix, and you should use StringBuilder
when String
concatenating inside of a loop. See the other answers for how this is done.
add a comment |
You are adding the value of two characters together. Change the String
concatenation from:
s += str.charAt(i) + str.charAt(i);
To:
s += str.charAt(i) + "" + str.charAt(i);
Which will ensure the characters convert to a String
.
Note: This is a quick fix, and you should use StringBuilder
when String
concatenating inside of a loop. See the other answers for how this is done.
add a comment |
You are adding the value of two characters together. Change the String
concatenation from:
s += str.charAt(i) + str.charAt(i);
To:
s += str.charAt(i) + "" + str.charAt(i);
Which will ensure the characters convert to a String
.
Note: This is a quick fix, and you should use StringBuilder
when String
concatenating inside of a loop. See the other answers for how this is done.
You are adding the value of two characters together. Change the String
concatenation from:
s += str.charAt(i) + str.charAt(i);
To:
s += str.charAt(i) + "" + str.charAt(i);
Which will ensure the characters convert to a String
.
Note: This is a quick fix, and you should use StringBuilder
when String
concatenating inside of a loop. See the other answers for how this is done.
edited 8 hours ago
answered 8 hours ago
NexevisNexevis
6801 silver badge11 bronze badges
6801 silver badge11 bronze badges
add a comment |
add a comment |
String.charAt()
returns a char
(https://docs.oracle.com/javase/7/docs/api/java/lang/String.html#charAt(int)) so you're dealing with a single character. With onechar
, you can perform operations on it like this (which will print "65" which is ASCII value for the 'A' character):
System.out.println('A' + 0);
you can print the ASCII value for the next character ("B") by adding 1 to 'A'
, like this:
System.out.println('A' + 1);
To make your code work – so that it doubles each character – there are number of options. You could append each character one at a time:
s += str.charAt(i);
s += str.charAt(i);
or various ways of casting the operation to a string:
s += "" + str.charAt(i) + str.charAt(i);
add a comment |
String.charAt()
returns a char
(https://docs.oracle.com/javase/7/docs/api/java/lang/String.html#charAt(int)) so you're dealing with a single character. With onechar
, you can perform operations on it like this (which will print "65" which is ASCII value for the 'A' character):
System.out.println('A' + 0);
you can print the ASCII value for the next character ("B") by adding 1 to 'A'
, like this:
System.out.println('A' + 1);
To make your code work – so that it doubles each character – there are number of options. You could append each character one at a time:
s += str.charAt(i);
s += str.charAt(i);
or various ways of casting the operation to a string:
s += "" + str.charAt(i) + str.charAt(i);
add a comment |
String.charAt()
returns a char
(https://docs.oracle.com/javase/7/docs/api/java/lang/String.html#charAt(int)) so you're dealing with a single character. With onechar
, you can perform operations on it like this (which will print "65" which is ASCII value for the 'A' character):
System.out.println('A' + 0);
you can print the ASCII value for the next character ("B") by adding 1 to 'A'
, like this:
System.out.println('A' + 1);
To make your code work – so that it doubles each character – there are number of options. You could append each character one at a time:
s += str.charAt(i);
s += str.charAt(i);
or various ways of casting the operation to a string:
s += "" + str.charAt(i) + str.charAt(i);
String.charAt()
returns a char
(https://docs.oracle.com/javase/7/docs/api/java/lang/String.html#charAt(int)) so you're dealing with a single character. With onechar
, you can perform operations on it like this (which will print "65" which is ASCII value for the 'A' character):
System.out.println('A' + 0);
you can print the ASCII value for the next character ("B") by adding 1 to 'A'
, like this:
System.out.println('A' + 1);
To make your code work – so that it doubles each character – there are number of options. You could append each character one at a time:
s += str.charAt(i);
s += str.charAt(i);
or various ways of casting the operation to a string:
s += "" + str.charAt(i) + str.charAt(i);
edited 6 hours ago
answered 8 hours ago
kaankaan
344 bronze badges
344 bronze badges
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f56977717%2fwhy-does-it-output-integers-instead-of-letters%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
IzU,hcFaiDeLvg3cj,qER,aDYWK VaCjhRlEsFBj,g9,wpqEH xal,6,s,OwabVlU6 lfegqXU82ONl4uE,u izcPfElVgSpJ,JfrPk3rF7Erh
5
Adding two
char
s doesn't give you a string with those two characters, but rather an int with numerical value equal to the sum of the two characters' numerical values.– Andrey Akhmetov
8 hours ago