Performance issue in code for reading line and testing for palindromeCheck if the string is palindromeCheck if a string is palindrome or two strings are the opposite of each otherTesting input integers for the palindrome propertyChecking for a palindromeMilliseconds to Time string & Time string to MillisecondsLongest Common Subsequence and Longest Subsequence PalindromeComparing a string using a stackCheck for Palindrome string in JavaLeetcode 125. Valid Palindrome (better performance?)Palindrome-testing Java program for an interviewPalindrome implementations - iterative and recursiveMatch a simple balanced language using a queue
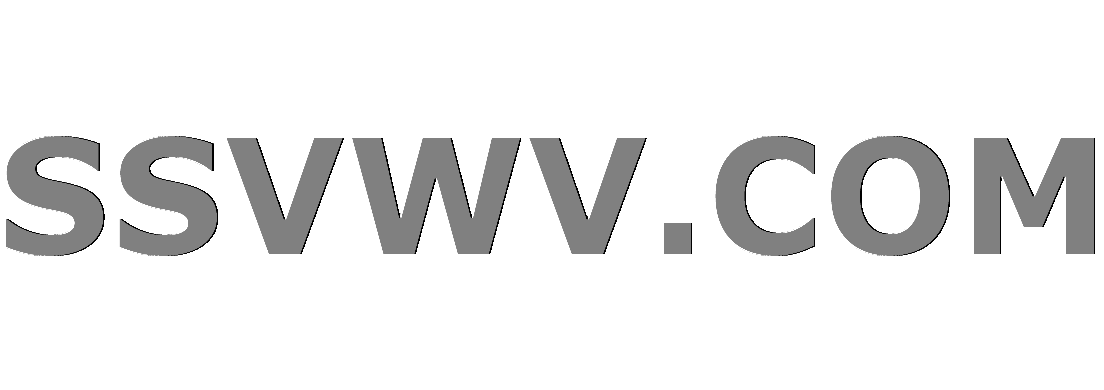
Multi tool use
Is it okay to use open source code to do an interview task?
How to evaluate the performance of open source solver?
What is a writing material that persists forever or for a long time?
Why was such an unrevealing title originally chosen and then changed for some International markets?
Optimization models for portfolio optimization
Did right-wing politician Franz Josef Strauss ever explain why he gave a 3 billion loan to East Germany in 1983?
VHDL: is there a way to create an entity into which constants can be passed?
Moving millions of files to a different directory with specfic name patterns
Did depressed people far more accurately estimate how many monsters they killed in a video game?
Writing an ace/aro character?
How to convert diagonal matrix to rectangular matrix
Is it possible for a character at any level to cast all 44 Cantrips in one week without Magic Items?
Would a Nikon FG 20 film SLR camera take pictures without batteries?
Is it possible to complete a PhD in CS in 3 years?
What exactly is a "murder hobo"?
Conditions for Roots of a quadratic equation at infinity
What was the profession 芸者 (female entertainer) called in Germany?
What are the effects of abstaining from eating a certain flavor?
Did Rabbi Akiva accept arguments from ignorance?
QR codes, do people use them?
What could cause the sea level to massively decrease?
Found and corrected a mistake on someone's else paper -- praxis?
Complementary transistor pair with a bipolar transistor and a MOSFET
Appropriate conduit for several data cables underground over 300' run
Performance issue in code for reading line and testing for palindrome
Check if the string is palindromeCheck if a string is palindrome or two strings are the opposite of each otherTesting input integers for the palindrome propertyChecking for a palindromeMilliseconds to Time string & Time string to MillisecondsLongest Common Subsequence and Longest Subsequence PalindromeComparing a string using a stackCheck for Palindrome string in JavaLeetcode 125. Valid Palindrome (better performance?)Palindrome-testing Java program for an interviewPalindrome implementations - iterative and recursiveMatch a simple balanced language using a queue
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty margin-bottom:0;
$begingroup$
This is some code that determines if a string of characters is a palindrome or not. My professor says that there is a performance issue with the program, but I can't quite put my finger on it. Can someone find out the 'performance' issue?
Initially, I thought maybe the process is slower as it uses two memory containers, as opposed to simply comparing two halves of a single string.
int main()
char c;
bool check = true;
stack<char> cstack;
queue<char> cqueue;
cout << "Enter a string and press return." << endl;
cin.get(c);
while (c != 'n')
cstack.push(c);
cqueue.push(c);
cin.get(c);
while (check && !cqueue.empty())
if (cstack.top() != cqueue.front())
check = false;
cstack.pop();
cqueue.pop();
if (check)
cout << "Yes it is!" << endl;
else
cout << "No it's not." << endl;
return 0;
c++ performance beginner strings palindrome
New contributor
Avantika P is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
add a comment |
$begingroup$
This is some code that determines if a string of characters is a palindrome or not. My professor says that there is a performance issue with the program, but I can't quite put my finger on it. Can someone find out the 'performance' issue?
Initially, I thought maybe the process is slower as it uses two memory containers, as opposed to simply comparing two halves of a single string.
int main()
char c;
bool check = true;
stack<char> cstack;
queue<char> cqueue;
cout << "Enter a string and press return." << endl;
cin.get(c);
while (c != 'n')
cstack.push(c);
cqueue.push(c);
cin.get(c);
while (check && !cqueue.empty())
if (cstack.top() != cqueue.front())
check = false;
cstack.pop();
cqueue.pop();
if (check)
cout << "Yes it is!" << endl;
else
cout << "No it's not." << endl;
return 0;
c++ performance beginner strings palindrome
New contributor
Avantika P is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
$begingroup$
Is there a way to combine stack top/pop and queue front/pop in a single statement?
$endgroup$
– dfhwze
7 hours ago
$begingroup$
You might want to have a look at this, though in that case the check was case insensitive.
$endgroup$
– Incomputable
4 hours ago
3
$begingroup$
As an aside, this is nearly a complete program. Consider giving the full program next time in a similar situation (changing this now is inadvisable).
$endgroup$
– Deduplicator
4 hours ago
add a comment |
$begingroup$
This is some code that determines if a string of characters is a palindrome or not. My professor says that there is a performance issue with the program, but I can't quite put my finger on it. Can someone find out the 'performance' issue?
Initially, I thought maybe the process is slower as it uses two memory containers, as opposed to simply comparing two halves of a single string.
int main()
char c;
bool check = true;
stack<char> cstack;
queue<char> cqueue;
cout << "Enter a string and press return." << endl;
cin.get(c);
while (c != 'n')
cstack.push(c);
cqueue.push(c);
cin.get(c);
while (check && !cqueue.empty())
if (cstack.top() != cqueue.front())
check = false;
cstack.pop();
cqueue.pop();
if (check)
cout << "Yes it is!" << endl;
else
cout << "No it's not." << endl;
return 0;
c++ performance beginner strings palindrome
New contributor
Avantika P is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
This is some code that determines if a string of characters is a palindrome or not. My professor says that there is a performance issue with the program, but I can't quite put my finger on it. Can someone find out the 'performance' issue?
Initially, I thought maybe the process is slower as it uses two memory containers, as opposed to simply comparing two halves of a single string.
int main()
char c;
bool check = true;
stack<char> cstack;
queue<char> cqueue;
cout << "Enter a string and press return." << endl;
cin.get(c);
while (c != 'n')
cstack.push(c);
cqueue.push(c);
cin.get(c);
while (check && !cqueue.empty())
if (cstack.top() != cqueue.front())
check = false;
cstack.pop();
cqueue.pop();
if (check)
cout << "Yes it is!" << endl;
else
cout << "No it's not." << endl;
return 0;
c++ performance beginner strings palindrome
c++ performance beginner strings palindrome
New contributor
Avantika P is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Avantika P is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
edited 4 hours ago
Deduplicator
12.9k20 silver badges52 bronze badges
12.9k20 silver badges52 bronze badges
New contributor
Avantika P is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked 8 hours ago


Avantika PAvantika P
162 bronze badges
162 bronze badges
New contributor
Avantika P is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Avantika P is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$begingroup$
Is there a way to combine stack top/pop and queue front/pop in a single statement?
$endgroup$
– dfhwze
7 hours ago
$begingroup$
You might want to have a look at this, though in that case the check was case insensitive.
$endgroup$
– Incomputable
4 hours ago
3
$begingroup$
As an aside, this is nearly a complete program. Consider giving the full program next time in a similar situation (changing this now is inadvisable).
$endgroup$
– Deduplicator
4 hours ago
add a comment |
$begingroup$
Is there a way to combine stack top/pop and queue front/pop in a single statement?
$endgroup$
– dfhwze
7 hours ago
$begingroup$
You might want to have a look at this, though in that case the check was case insensitive.
$endgroup$
– Incomputable
4 hours ago
3
$begingroup$
As an aside, this is nearly a complete program. Consider giving the full program next time in a similar situation (changing this now is inadvisable).
$endgroup$
– Deduplicator
4 hours ago
$begingroup$
Is there a way to combine stack top/pop and queue front/pop in a single statement?
$endgroup$
– dfhwze
7 hours ago
$begingroup$
Is there a way to combine stack top/pop and queue front/pop in a single statement?
$endgroup$
– dfhwze
7 hours ago
$begingroup$
You might want to have a look at this, though in that case the check was case insensitive.
$endgroup$
– Incomputable
4 hours ago
$begingroup$
You might want to have a look at this, though in that case the check was case insensitive.
$endgroup$
– Incomputable
4 hours ago
3
3
$begingroup$
As an aside, this is nearly a complete program. Consider giving the full program next time in a similar situation (changing this now is inadvisable).
$endgroup$
– Deduplicator
4 hours ago
$begingroup$
As an aside, this is nearly a complete program. Consider giving the full program next time in a similar situation (changing this now is inadvisable).
$endgroup$
– Deduplicator
4 hours ago
add a comment |
2 Answers
2
active
oldest
votes
$begingroup$
While it is not quite definitive, it looks like you use
using namespace std;
.
That namespace is not designed for wholesale inclusion, being vast and subject to change at the whim of the implementation, aside from providing what is standardised.
Read "Why is “using namespace std” considered bad practice?" for more detail.You should desist from using
std::endl
, as spurious manual flushing flushes any pretense at performance down the drain.
For those rare cases where it is actually necessary for correctness, usestd::flush
for explicitness.You assume reading from
std::cin
always succeeds. That's generally unsupportable, please handle failure gracefully.You are reading character-by-character. Each and every read has significant overhead, which you could simply avoid by using
std::getline()
. Using the proper abstraction is also significantly more readable.You are storing the input twice, once in a
std::queue
and once in astd::stack
. Even only storing it in just onestd::deque
(the underlying implementation for both) would be a considerable improvement.Consider encapsulating the test whether the input is a palindrome into its own reusable function, separate from actually getting it.
Testing whether something is a palindrome seems a favorite passtime of many beginners.
Thus, there are a myriad posts on how to efficiently and elegantly do that in C++, for example "Check if a string is palindrome or two strings are the opposite of each other".
The important points are avoiding expensive copies, and only comparing each element once.If you want one of two values, conditional on some expression, consider the conditional operator
expr ? true_expr : false_expr
. It is designed for that.return 0;
is implicit formain()
. Make of that what you will.
$endgroup$
add a comment |
$begingroup$
I see two improvement points in the code.
- It is better to use getLine() and store the input in char* instead of reading each char and appending to a stack
- It is more than enough to iterate till half of the string as the remaining half is checked in the first half iteration
cstack.top() != cqueue.front()
$endgroup$
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "196"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Avantika P is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f223680%2fperformance-issue-in-code-for-reading-line-and-testing-for-palindrome%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
$begingroup$
While it is not quite definitive, it looks like you use
using namespace std;
.
That namespace is not designed for wholesale inclusion, being vast and subject to change at the whim of the implementation, aside from providing what is standardised.
Read "Why is “using namespace std” considered bad practice?" for more detail.You should desist from using
std::endl
, as spurious manual flushing flushes any pretense at performance down the drain.
For those rare cases where it is actually necessary for correctness, usestd::flush
for explicitness.You assume reading from
std::cin
always succeeds. That's generally unsupportable, please handle failure gracefully.You are reading character-by-character. Each and every read has significant overhead, which you could simply avoid by using
std::getline()
. Using the proper abstraction is also significantly more readable.You are storing the input twice, once in a
std::queue
and once in astd::stack
. Even only storing it in just onestd::deque
(the underlying implementation for both) would be a considerable improvement.Consider encapsulating the test whether the input is a palindrome into its own reusable function, separate from actually getting it.
Testing whether something is a palindrome seems a favorite passtime of many beginners.
Thus, there are a myriad posts on how to efficiently and elegantly do that in C++, for example "Check if a string is palindrome or two strings are the opposite of each other".
The important points are avoiding expensive copies, and only comparing each element once.If you want one of two values, conditional on some expression, consider the conditional operator
expr ? true_expr : false_expr
. It is designed for that.return 0;
is implicit formain()
. Make of that what you will.
$endgroup$
add a comment |
$begingroup$
While it is not quite definitive, it looks like you use
using namespace std;
.
That namespace is not designed for wholesale inclusion, being vast and subject to change at the whim of the implementation, aside from providing what is standardised.
Read "Why is “using namespace std” considered bad practice?" for more detail.You should desist from using
std::endl
, as spurious manual flushing flushes any pretense at performance down the drain.
For those rare cases where it is actually necessary for correctness, usestd::flush
for explicitness.You assume reading from
std::cin
always succeeds. That's generally unsupportable, please handle failure gracefully.You are reading character-by-character. Each and every read has significant overhead, which you could simply avoid by using
std::getline()
. Using the proper abstraction is also significantly more readable.You are storing the input twice, once in a
std::queue
and once in astd::stack
. Even only storing it in just onestd::deque
(the underlying implementation for both) would be a considerable improvement.Consider encapsulating the test whether the input is a palindrome into its own reusable function, separate from actually getting it.
Testing whether something is a palindrome seems a favorite passtime of many beginners.
Thus, there are a myriad posts on how to efficiently and elegantly do that in C++, for example "Check if a string is palindrome or two strings are the opposite of each other".
The important points are avoiding expensive copies, and only comparing each element once.If you want one of two values, conditional on some expression, consider the conditional operator
expr ? true_expr : false_expr
. It is designed for that.return 0;
is implicit formain()
. Make of that what you will.
$endgroup$
add a comment |
$begingroup$
While it is not quite definitive, it looks like you use
using namespace std;
.
That namespace is not designed for wholesale inclusion, being vast and subject to change at the whim of the implementation, aside from providing what is standardised.
Read "Why is “using namespace std” considered bad practice?" for more detail.You should desist from using
std::endl
, as spurious manual flushing flushes any pretense at performance down the drain.
For those rare cases where it is actually necessary for correctness, usestd::flush
for explicitness.You assume reading from
std::cin
always succeeds. That's generally unsupportable, please handle failure gracefully.You are reading character-by-character. Each and every read has significant overhead, which you could simply avoid by using
std::getline()
. Using the proper abstraction is also significantly more readable.You are storing the input twice, once in a
std::queue
and once in astd::stack
. Even only storing it in just onestd::deque
(the underlying implementation for both) would be a considerable improvement.Consider encapsulating the test whether the input is a palindrome into its own reusable function, separate from actually getting it.
Testing whether something is a palindrome seems a favorite passtime of many beginners.
Thus, there are a myriad posts on how to efficiently and elegantly do that in C++, for example "Check if a string is palindrome or two strings are the opposite of each other".
The important points are avoiding expensive copies, and only comparing each element once.If you want one of two values, conditional on some expression, consider the conditional operator
expr ? true_expr : false_expr
. It is designed for that.return 0;
is implicit formain()
. Make of that what you will.
$endgroup$
While it is not quite definitive, it looks like you use
using namespace std;
.
That namespace is not designed for wholesale inclusion, being vast and subject to change at the whim of the implementation, aside from providing what is standardised.
Read "Why is “using namespace std” considered bad practice?" for more detail.You should desist from using
std::endl
, as spurious manual flushing flushes any pretense at performance down the drain.
For those rare cases where it is actually necessary for correctness, usestd::flush
for explicitness.You assume reading from
std::cin
always succeeds. That's generally unsupportable, please handle failure gracefully.You are reading character-by-character. Each and every read has significant overhead, which you could simply avoid by using
std::getline()
. Using the proper abstraction is also significantly more readable.You are storing the input twice, once in a
std::queue
and once in astd::stack
. Even only storing it in just onestd::deque
(the underlying implementation for both) would be a considerable improvement.Consider encapsulating the test whether the input is a palindrome into its own reusable function, separate from actually getting it.
Testing whether something is a palindrome seems a favorite passtime of many beginners.
Thus, there are a myriad posts on how to efficiently and elegantly do that in C++, for example "Check if a string is palindrome or two strings are the opposite of each other".
The important points are avoiding expensive copies, and only comparing each element once.If you want one of two values, conditional on some expression, consider the conditional operator
expr ? true_expr : false_expr
. It is designed for that.return 0;
is implicit formain()
. Make of that what you will.
answered 4 hours ago
DeduplicatorDeduplicator
12.9k20 silver badges52 bronze badges
12.9k20 silver badges52 bronze badges
add a comment |
add a comment |
$begingroup$
I see two improvement points in the code.
- It is better to use getLine() and store the input in char* instead of reading each char and appending to a stack
- It is more than enough to iterate till half of the string as the remaining half is checked in the first half iteration
cstack.top() != cqueue.front()
$endgroup$
add a comment |
$begingroup$
I see two improvement points in the code.
- It is better to use getLine() and store the input in char* instead of reading each char and appending to a stack
- It is more than enough to iterate till half of the string as the remaining half is checked in the first half iteration
cstack.top() != cqueue.front()
$endgroup$
add a comment |
$begingroup$
I see two improvement points in the code.
- It is better to use getLine() and store the input in char* instead of reading each char and appending to a stack
- It is more than enough to iterate till half of the string as the remaining half is checked in the first half iteration
cstack.top() != cqueue.front()
$endgroup$
I see two improvement points in the code.
- It is better to use getLine() and store the input in char* instead of reading each char and appending to a stack
- It is more than enough to iterate till half of the string as the remaining half is checked in the first half iteration
cstack.top() != cqueue.front()
answered 7 hours ago
Ramanathan GanesanRamanathan Ganesan
4394 silver badges5 bronze badges
4394 silver badges5 bronze badges
add a comment |
add a comment |
Avantika P is a new contributor. Be nice, and check out our Code of Conduct.
Avantika P is a new contributor. Be nice, and check out our Code of Conduct.
Avantika P is a new contributor. Be nice, and check out our Code of Conduct.
Avantika P is a new contributor. Be nice, and check out our Code of Conduct.
Thanks for contributing an answer to Code Review Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f223680%2fperformance-issue-in-code-for-reading-line-and-testing-for-palindrome%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
G Rkp,C tBBOzDnOchUGtg1rbc,LIxJSA0M4Kz9GS6US7BvPzi
$begingroup$
Is there a way to combine stack top/pop and queue front/pop in a single statement?
$endgroup$
– dfhwze
7 hours ago
$begingroup$
You might want to have a look at this, though in that case the check was case insensitive.
$endgroup$
– Incomputable
4 hours ago
3
$begingroup$
As an aside, this is nearly a complete program. Consider giving the full program next time in a similar situation (changing this now is inadvisable).
$endgroup$
– Deduplicator
4 hours ago