Defining a function which returns a function pointer which also returns a function pointer without typedefsHow do function pointers in C work?Understanding typedefs for function pointers in CTypedef function pointer?Returning function pointer typetypedef function pointers and extern keywordError with function pointer returning a (void *)Passing capturing lambda as function pointerPass typedefed function pointerReturn a function pointer without a typedef
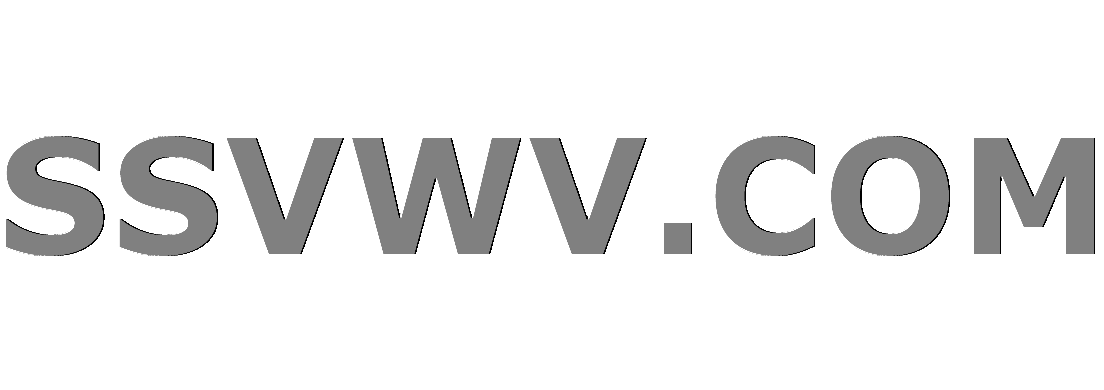
Multi tool use
Will replacing a fake visa with a different fake visa cause me problems when applying for a legal study permit?
Georgian capital letter “Ⴒ” (“tar”) in pdfLaTeX
Are the definite and indefinite integrals actually two different things? Where is the flaw in my understanding?
Why do sellers care about down payments?
How does Vivi differ from other Black Mages?
Is there a star over my head?
Evidence that matrix multiplication cannot be done in O(n^2 poly(log(n))) time
Kerning feedback on logo
Random point on a sphere
Can a new chain significantly improve the riding experience? If yes - what else can?
Are Democrats more likely to believe Astrology is a science?
Why the word "rain" is considered a verb if it is not possible to conjugate it?
Exact Brexit date and consequences
How is the Team Scooby Doo funded?
Why should I always enable compiler warnings?
Gas pipes - why does gas burn "outwards?"
If you have multiple situational racial save bonuses and are in a situation where they all apply do they stack?
Might have gotten a coworker sick, should I address this?
Do ibuprofen or paracetamol cause hearing loss?
extract lines from bottom until regex match
Does the amount of +1/+1 from *prowess* remain on a creature, even when a creature gets flipped face-down by Ixidron?
Why does F + F' = 1?
Seized engine due to being run without oil
What does "synoptic" mean in avionics?
Defining a function which returns a function pointer which also returns a function pointer without typedefs
How do function pointers in C work?Understanding typedefs for function pointers in CTypedef function pointer?Returning function pointer typetypedef function pointers and extern keywordError with function pointer returning a (void *)Passing capturing lambda as function pointerPass typedefed function pointerReturn a function pointer without a typedef
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty margin-bottom:0;
I am trying to really understand function pointers without using typedef
but cannot seem to get this. I do not understand what signature is needed to convey that I return a pointer to a pointer to a function.
#include <stdio.h>
void odd() printf("odd!n");
void even() printf("even!n");
void (*get_pfn(int i))()
return i % 2 == 0 ? &even : &odd;
__SIGNATURE__
return &get_pfn;
int main()
get_pfn_pfn()(1)();
get_pfn_pfn()(2)();
return 0;
To make this work, what does __SIGNATURE__
have to be?
c function function-pointers
add a comment |
I am trying to really understand function pointers without using typedef
but cannot seem to get this. I do not understand what signature is needed to convey that I return a pointer to a pointer to a function.
#include <stdio.h>
void odd() printf("odd!n");
void even() printf("even!n");
void (*get_pfn(int i))()
return i % 2 == 0 ? &even : &odd;
__SIGNATURE__
return &get_pfn;
int main()
get_pfn_pfn()(1)();
get_pfn_pfn()(2)();
return 0;
To make this work, what does __SIGNATURE__
have to be?
c function function-pointers
5
Good question - now don't ever do this again. :)
– Michael Dorgan
10 hours ago
5
use cdecl
– Antti Haapala
9 hours ago
add a comment |
I am trying to really understand function pointers without using typedef
but cannot seem to get this. I do not understand what signature is needed to convey that I return a pointer to a pointer to a function.
#include <stdio.h>
void odd() printf("odd!n");
void even() printf("even!n");
void (*get_pfn(int i))()
return i % 2 == 0 ? &even : &odd;
__SIGNATURE__
return &get_pfn;
int main()
get_pfn_pfn()(1)();
get_pfn_pfn()(2)();
return 0;
To make this work, what does __SIGNATURE__
have to be?
c function function-pointers
I am trying to really understand function pointers without using typedef
but cannot seem to get this. I do not understand what signature is needed to convey that I return a pointer to a pointer to a function.
#include <stdio.h>
void odd() printf("odd!n");
void even() printf("even!n");
void (*get_pfn(int i))()
return i % 2 == 0 ? &even : &odd;
__SIGNATURE__
return &get_pfn;
int main()
get_pfn_pfn()(1)();
get_pfn_pfn()(2)();
return 0;
To make this work, what does __SIGNATURE__
have to be?
c function function-pointers
c function function-pointers
edited 1 hour ago


Justin
15.3k10 gold badges63 silver badges106 bronze badges
15.3k10 gold badges63 silver badges106 bronze badges
asked 10 hours ago
JasJas
5095 silver badges14 bronze badges
5095 silver badges14 bronze badges
5
Good question - now don't ever do this again. :)
– Michael Dorgan
10 hours ago
5
use cdecl
– Antti Haapala
9 hours ago
add a comment |
5
Good question - now don't ever do this again. :)
– Michael Dorgan
10 hours ago
5
use cdecl
– Antti Haapala
9 hours ago
5
5
Good question - now don't ever do this again. :)
– Michael Dorgan
10 hours ago
Good question - now don't ever do this again. :)
– Michael Dorgan
10 hours ago
5
5
use cdecl
– Antti Haapala
9 hours ago
use cdecl
– Antti Haapala
9 hours ago
add a comment |
4 Answers
4
active
oldest
votes
It has to return a function pointer to a function that takes an int
and returns a function pointer:
void (*(*get_pfn_pfn(void))(int))(void)
return &get_pfn;
more lines:
void (*
(*
get_pfn_pfn(void) // this is our function
)(int i) // this is get_pfn(int)
)(void) // this is odd() or even()
return &get_pfn;
The void
s can be omitted, in which case the function pointer points to a function that takes unknown number of parameters. Which is not what you want. To declare a function pointer to a function that takes no arguments, you should add void
inside function parameter list. The same way it's best to change get_pfn
to void (*get_pfn(int i))(void)
. For example try calling from get_pfn(1)("some arg", "some other arg");
. A C compiler will not give a warning, as empty ()
denote unknown arguments. To say that function takes no arguments, you have to (void)
.
For many the sequences of braces, especially ))(
, in function pointers are hard to parse. That's why many prefer typedefs for function pointers or types:
typedef void get_pfn_func_t(void);
get_pfn_func_t *get_pfn(int i)
return i % 2 == 0 ? &even : &odd;
typedef get_pfn_func_t *get_pfn_pfn_func_t(int i);
get_pfn_pfn_func_t *get_pfn_pfn(void)
return &get_pfn;
2
This one confirmed to work with online C check.
– Michael Dorgan
10 hours ago
Thank you so so much Kamil!! I like that broken down/visualized tree like view, it helped make it a lot more understandable :) !!
– Jas
10 hours ago
1
@Jas You may like it but I don't think it is recommended to write code in this manner.
– machine_1
10 hours ago
I wish there was a website that had a GUI representation of a function pointer, let you move various components around, etc. Something like nsdateformatter.com
– Alexander
1 hour ago
add a comment |
The return type of the function get_pfn
is -
void (*) ();
So type of &get_pfn
is -
void (*(*)(int))()
Now, this function returns this type, hence it's signature will be -
void (*(*(foo)())(int))()
You can verify this by typing this in cdecl.org
Thank you very much Ajay! Your explanation breaking down the steps helped me understand it more easily, but unfortunately the signature did not work sorry :(
– Jas
10 hours ago
1
@Jas, sorry I messed up the order of the(int)
. I thought your odd even functions take a param int. I will correct it.
– Ajay Brahmakshatriya
10 hours ago
1
@Jas, I have corrected my answer,
– Ajay Brahmakshatriya
10 hours ago
add a comment |
Function pointers without a typedef
can be tricky to work with. To figure them out, you work from the inside out.
So let's break down exactly how we come up with the correct function signature.
get_pfn_pfn
is a function:
get_pfn_pfn()
Which takes no parameters:
get_pfn_pfn(void)
And returns a pointer:
*get_pfn_pfn(void)
To a function:
(*get_pfn_pfn(void))()
Which takes an int
parameter:
(*get_pfn_pfn(void))(int)
And returns a pointer:
*(*get_pfn_pfn(void))(int)
To a function:
(*(*get_pfn_pfn(void))(int))()
Which takes no parameters:
(*(*get_pfn_pfn(void))(int))(void)
And returns nothing (i.e. void
):
void (*(*get_pfn_pfn(void))(int))(void)
Of course, using typedef
's simplifies this greatly.
First the type for even
and odd
:
typedef void (*func1)(void);
Which we can then apply to get_pfn
:
func1 get_pfn(int) ...
Then the type for this function:
typedef func1 (*func2)(int);
Which we can apply to get_pfn_pfn
:
func2 get_pfn_pfn(void) ...
add a comment |
it's this way:
void (*(*get_pfn_pfn(void))(int))()
New contributor
Abdelfettah Besbes is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/4.0/"u003ecc by-sa 4.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f57875417%2fdefining-a-function-which-returns-a-function-pointer-which-also-returns-a-functi%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
It has to return a function pointer to a function that takes an int
and returns a function pointer:
void (*(*get_pfn_pfn(void))(int))(void)
return &get_pfn;
more lines:
void (*
(*
get_pfn_pfn(void) // this is our function
)(int i) // this is get_pfn(int)
)(void) // this is odd() or even()
return &get_pfn;
The void
s can be omitted, in which case the function pointer points to a function that takes unknown number of parameters. Which is not what you want. To declare a function pointer to a function that takes no arguments, you should add void
inside function parameter list. The same way it's best to change get_pfn
to void (*get_pfn(int i))(void)
. For example try calling from get_pfn(1)("some arg", "some other arg");
. A C compiler will not give a warning, as empty ()
denote unknown arguments. To say that function takes no arguments, you have to (void)
.
For many the sequences of braces, especially ))(
, in function pointers are hard to parse. That's why many prefer typedefs for function pointers or types:
typedef void get_pfn_func_t(void);
get_pfn_func_t *get_pfn(int i)
return i % 2 == 0 ? &even : &odd;
typedef get_pfn_func_t *get_pfn_pfn_func_t(int i);
get_pfn_pfn_func_t *get_pfn_pfn(void)
return &get_pfn;
2
This one confirmed to work with online C check.
– Michael Dorgan
10 hours ago
Thank you so so much Kamil!! I like that broken down/visualized tree like view, it helped make it a lot more understandable :) !!
– Jas
10 hours ago
1
@Jas You may like it but I don't think it is recommended to write code in this manner.
– machine_1
10 hours ago
I wish there was a website that had a GUI representation of a function pointer, let you move various components around, etc. Something like nsdateformatter.com
– Alexander
1 hour ago
add a comment |
It has to return a function pointer to a function that takes an int
and returns a function pointer:
void (*(*get_pfn_pfn(void))(int))(void)
return &get_pfn;
more lines:
void (*
(*
get_pfn_pfn(void) // this is our function
)(int i) // this is get_pfn(int)
)(void) // this is odd() or even()
return &get_pfn;
The void
s can be omitted, in which case the function pointer points to a function that takes unknown number of parameters. Which is not what you want. To declare a function pointer to a function that takes no arguments, you should add void
inside function parameter list. The same way it's best to change get_pfn
to void (*get_pfn(int i))(void)
. For example try calling from get_pfn(1)("some arg", "some other arg");
. A C compiler will not give a warning, as empty ()
denote unknown arguments. To say that function takes no arguments, you have to (void)
.
For many the sequences of braces, especially ))(
, in function pointers are hard to parse. That's why many prefer typedefs for function pointers or types:
typedef void get_pfn_func_t(void);
get_pfn_func_t *get_pfn(int i)
return i % 2 == 0 ? &even : &odd;
typedef get_pfn_func_t *get_pfn_pfn_func_t(int i);
get_pfn_pfn_func_t *get_pfn_pfn(void)
return &get_pfn;
2
This one confirmed to work with online C check.
– Michael Dorgan
10 hours ago
Thank you so so much Kamil!! I like that broken down/visualized tree like view, it helped make it a lot more understandable :) !!
– Jas
10 hours ago
1
@Jas You may like it but I don't think it is recommended to write code in this manner.
– machine_1
10 hours ago
I wish there was a website that had a GUI representation of a function pointer, let you move various components around, etc. Something like nsdateformatter.com
– Alexander
1 hour ago
add a comment |
It has to return a function pointer to a function that takes an int
and returns a function pointer:
void (*(*get_pfn_pfn(void))(int))(void)
return &get_pfn;
more lines:
void (*
(*
get_pfn_pfn(void) // this is our function
)(int i) // this is get_pfn(int)
)(void) // this is odd() or even()
return &get_pfn;
The void
s can be omitted, in which case the function pointer points to a function that takes unknown number of parameters. Which is not what you want. To declare a function pointer to a function that takes no arguments, you should add void
inside function parameter list. The same way it's best to change get_pfn
to void (*get_pfn(int i))(void)
. For example try calling from get_pfn(1)("some arg", "some other arg");
. A C compiler will not give a warning, as empty ()
denote unknown arguments. To say that function takes no arguments, you have to (void)
.
For many the sequences of braces, especially ))(
, in function pointers are hard to parse. That's why many prefer typedefs for function pointers or types:
typedef void get_pfn_func_t(void);
get_pfn_func_t *get_pfn(int i)
return i % 2 == 0 ? &even : &odd;
typedef get_pfn_func_t *get_pfn_pfn_func_t(int i);
get_pfn_pfn_func_t *get_pfn_pfn(void)
return &get_pfn;
It has to return a function pointer to a function that takes an int
and returns a function pointer:
void (*(*get_pfn_pfn(void))(int))(void)
return &get_pfn;
more lines:
void (*
(*
get_pfn_pfn(void) // this is our function
)(int i) // this is get_pfn(int)
)(void) // this is odd() or even()
return &get_pfn;
The void
s can be omitted, in which case the function pointer points to a function that takes unknown number of parameters. Which is not what you want. To declare a function pointer to a function that takes no arguments, you should add void
inside function parameter list. The same way it's best to change get_pfn
to void (*get_pfn(int i))(void)
. For example try calling from get_pfn(1)("some arg", "some other arg");
. A C compiler will not give a warning, as empty ()
denote unknown arguments. To say that function takes no arguments, you have to (void)
.
For many the sequences of braces, especially ))(
, in function pointers are hard to parse. That's why many prefer typedefs for function pointers or types:
typedef void get_pfn_func_t(void);
get_pfn_func_t *get_pfn(int i)
return i % 2 == 0 ? &even : &odd;
typedef get_pfn_func_t *get_pfn_pfn_func_t(int i);
get_pfn_pfn_func_t *get_pfn_pfn(void)
return &get_pfn;
edited 10 hours ago
answered 10 hours ago


Kamil CukKamil Cuk
21.5k2 gold badges9 silver badges37 bronze badges
21.5k2 gold badges9 silver badges37 bronze badges
2
This one confirmed to work with online C check.
– Michael Dorgan
10 hours ago
Thank you so so much Kamil!! I like that broken down/visualized tree like view, it helped make it a lot more understandable :) !!
– Jas
10 hours ago
1
@Jas You may like it but I don't think it is recommended to write code in this manner.
– machine_1
10 hours ago
I wish there was a website that had a GUI representation of a function pointer, let you move various components around, etc. Something like nsdateformatter.com
– Alexander
1 hour ago
add a comment |
2
This one confirmed to work with online C check.
– Michael Dorgan
10 hours ago
Thank you so so much Kamil!! I like that broken down/visualized tree like view, it helped make it a lot more understandable :) !!
– Jas
10 hours ago
1
@Jas You may like it but I don't think it is recommended to write code in this manner.
– machine_1
10 hours ago
I wish there was a website that had a GUI representation of a function pointer, let you move various components around, etc. Something like nsdateformatter.com
– Alexander
1 hour ago
2
2
This one confirmed to work with online C check.
– Michael Dorgan
10 hours ago
This one confirmed to work with online C check.
– Michael Dorgan
10 hours ago
Thank you so so much Kamil!! I like that broken down/visualized tree like view, it helped make it a lot more understandable :) !!
– Jas
10 hours ago
Thank you so so much Kamil!! I like that broken down/visualized tree like view, it helped make it a lot more understandable :) !!
– Jas
10 hours ago
1
1
@Jas You may like it but I don't think it is recommended to write code in this manner.
– machine_1
10 hours ago
@Jas You may like it but I don't think it is recommended to write code in this manner.
– machine_1
10 hours ago
I wish there was a website that had a GUI representation of a function pointer, let you move various components around, etc. Something like nsdateformatter.com
– Alexander
1 hour ago
I wish there was a website that had a GUI representation of a function pointer, let you move various components around, etc. Something like nsdateformatter.com
– Alexander
1 hour ago
add a comment |
The return type of the function get_pfn
is -
void (*) ();
So type of &get_pfn
is -
void (*(*)(int))()
Now, this function returns this type, hence it's signature will be -
void (*(*(foo)())(int))()
You can verify this by typing this in cdecl.org
Thank you very much Ajay! Your explanation breaking down the steps helped me understand it more easily, but unfortunately the signature did not work sorry :(
– Jas
10 hours ago
1
@Jas, sorry I messed up the order of the(int)
. I thought your odd even functions take a param int. I will correct it.
– Ajay Brahmakshatriya
10 hours ago
1
@Jas, I have corrected my answer,
– Ajay Brahmakshatriya
10 hours ago
add a comment |
The return type of the function get_pfn
is -
void (*) ();
So type of &get_pfn
is -
void (*(*)(int))()
Now, this function returns this type, hence it's signature will be -
void (*(*(foo)())(int))()
You can verify this by typing this in cdecl.org
Thank you very much Ajay! Your explanation breaking down the steps helped me understand it more easily, but unfortunately the signature did not work sorry :(
– Jas
10 hours ago
1
@Jas, sorry I messed up the order of the(int)
. I thought your odd even functions take a param int. I will correct it.
– Ajay Brahmakshatriya
10 hours ago
1
@Jas, I have corrected my answer,
– Ajay Brahmakshatriya
10 hours ago
add a comment |
The return type of the function get_pfn
is -
void (*) ();
So type of &get_pfn
is -
void (*(*)(int))()
Now, this function returns this type, hence it's signature will be -
void (*(*(foo)())(int))()
You can verify this by typing this in cdecl.org
The return type of the function get_pfn
is -
void (*) ();
So type of &get_pfn
is -
void (*(*)(int))()
Now, this function returns this type, hence it's signature will be -
void (*(*(foo)())(int))()
You can verify this by typing this in cdecl.org
edited 10 hours ago
answered 10 hours ago


Ajay BrahmakshatriyaAjay Brahmakshatriya
7,2453 gold badges16 silver badges36 bronze badges
7,2453 gold badges16 silver badges36 bronze badges
Thank you very much Ajay! Your explanation breaking down the steps helped me understand it more easily, but unfortunately the signature did not work sorry :(
– Jas
10 hours ago
1
@Jas, sorry I messed up the order of the(int)
. I thought your odd even functions take a param int. I will correct it.
– Ajay Brahmakshatriya
10 hours ago
1
@Jas, I have corrected my answer,
– Ajay Brahmakshatriya
10 hours ago
add a comment |
Thank you very much Ajay! Your explanation breaking down the steps helped me understand it more easily, but unfortunately the signature did not work sorry :(
– Jas
10 hours ago
1
@Jas, sorry I messed up the order of the(int)
. I thought your odd even functions take a param int. I will correct it.
– Ajay Brahmakshatriya
10 hours ago
1
@Jas, I have corrected my answer,
– Ajay Brahmakshatriya
10 hours ago
Thank you very much Ajay! Your explanation breaking down the steps helped me understand it more easily, but unfortunately the signature did not work sorry :(
– Jas
10 hours ago
Thank you very much Ajay! Your explanation breaking down the steps helped me understand it more easily, but unfortunately the signature did not work sorry :(
– Jas
10 hours ago
1
1
@Jas, sorry I messed up the order of the
(int)
. I thought your odd even functions take a param int. I will correct it.– Ajay Brahmakshatriya
10 hours ago
@Jas, sorry I messed up the order of the
(int)
. I thought your odd even functions take a param int. I will correct it.– Ajay Brahmakshatriya
10 hours ago
1
1
@Jas, I have corrected my answer,
– Ajay Brahmakshatriya
10 hours ago
@Jas, I have corrected my answer,
– Ajay Brahmakshatriya
10 hours ago
add a comment |
Function pointers without a typedef
can be tricky to work with. To figure them out, you work from the inside out.
So let's break down exactly how we come up with the correct function signature.
get_pfn_pfn
is a function:
get_pfn_pfn()
Which takes no parameters:
get_pfn_pfn(void)
And returns a pointer:
*get_pfn_pfn(void)
To a function:
(*get_pfn_pfn(void))()
Which takes an int
parameter:
(*get_pfn_pfn(void))(int)
And returns a pointer:
*(*get_pfn_pfn(void))(int)
To a function:
(*(*get_pfn_pfn(void))(int))()
Which takes no parameters:
(*(*get_pfn_pfn(void))(int))(void)
And returns nothing (i.e. void
):
void (*(*get_pfn_pfn(void))(int))(void)
Of course, using typedef
's simplifies this greatly.
First the type for even
and odd
:
typedef void (*func1)(void);
Which we can then apply to get_pfn
:
func1 get_pfn(int) ...
Then the type for this function:
typedef func1 (*func2)(int);
Which we can apply to get_pfn_pfn
:
func2 get_pfn_pfn(void) ...
add a comment |
Function pointers without a typedef
can be tricky to work with. To figure them out, you work from the inside out.
So let's break down exactly how we come up with the correct function signature.
get_pfn_pfn
is a function:
get_pfn_pfn()
Which takes no parameters:
get_pfn_pfn(void)
And returns a pointer:
*get_pfn_pfn(void)
To a function:
(*get_pfn_pfn(void))()
Which takes an int
parameter:
(*get_pfn_pfn(void))(int)
And returns a pointer:
*(*get_pfn_pfn(void))(int)
To a function:
(*(*get_pfn_pfn(void))(int))()
Which takes no parameters:
(*(*get_pfn_pfn(void))(int))(void)
And returns nothing (i.e. void
):
void (*(*get_pfn_pfn(void))(int))(void)
Of course, using typedef
's simplifies this greatly.
First the type for even
and odd
:
typedef void (*func1)(void);
Which we can then apply to get_pfn
:
func1 get_pfn(int) ...
Then the type for this function:
typedef func1 (*func2)(int);
Which we can apply to get_pfn_pfn
:
func2 get_pfn_pfn(void) ...
add a comment |
Function pointers without a typedef
can be tricky to work with. To figure them out, you work from the inside out.
So let's break down exactly how we come up with the correct function signature.
get_pfn_pfn
is a function:
get_pfn_pfn()
Which takes no parameters:
get_pfn_pfn(void)
And returns a pointer:
*get_pfn_pfn(void)
To a function:
(*get_pfn_pfn(void))()
Which takes an int
parameter:
(*get_pfn_pfn(void))(int)
And returns a pointer:
*(*get_pfn_pfn(void))(int)
To a function:
(*(*get_pfn_pfn(void))(int))()
Which takes no parameters:
(*(*get_pfn_pfn(void))(int))(void)
And returns nothing (i.e. void
):
void (*(*get_pfn_pfn(void))(int))(void)
Of course, using typedef
's simplifies this greatly.
First the type for even
and odd
:
typedef void (*func1)(void);
Which we can then apply to get_pfn
:
func1 get_pfn(int) ...
Then the type for this function:
typedef func1 (*func2)(int);
Which we can apply to get_pfn_pfn
:
func2 get_pfn_pfn(void) ...
Function pointers without a typedef
can be tricky to work with. To figure them out, you work from the inside out.
So let's break down exactly how we come up with the correct function signature.
get_pfn_pfn
is a function:
get_pfn_pfn()
Which takes no parameters:
get_pfn_pfn(void)
And returns a pointer:
*get_pfn_pfn(void)
To a function:
(*get_pfn_pfn(void))()
Which takes an int
parameter:
(*get_pfn_pfn(void))(int)
And returns a pointer:
*(*get_pfn_pfn(void))(int)
To a function:
(*(*get_pfn_pfn(void))(int))()
Which takes no parameters:
(*(*get_pfn_pfn(void))(int))(void)
And returns nothing (i.e. void
):
void (*(*get_pfn_pfn(void))(int))(void)
Of course, using typedef
's simplifies this greatly.
First the type for even
and odd
:
typedef void (*func1)(void);
Which we can then apply to get_pfn
:
func1 get_pfn(int) ...
Then the type for this function:
typedef func1 (*func2)(int);
Which we can apply to get_pfn_pfn
:
func2 get_pfn_pfn(void) ...
edited 10 hours ago
answered 10 hours ago
dbushdbush
112k15 gold badges127 silver badges159 bronze badges
112k15 gold badges127 silver badges159 bronze badges
add a comment |
add a comment |
it's this way:
void (*(*get_pfn_pfn(void))(int))()
New contributor
Abdelfettah Besbes is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
it's this way:
void (*(*get_pfn_pfn(void))(int))()
New contributor
Abdelfettah Besbes is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
it's this way:
void (*(*get_pfn_pfn(void))(int))()
New contributor
Abdelfettah Besbes is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
it's this way:
void (*(*get_pfn_pfn(void))(int))()
New contributor
Abdelfettah Besbes is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
edited 10 hours ago
New contributor
Abdelfettah Besbes is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
answered 10 hours ago
Abdelfettah BesbesAbdelfettah Besbes
31110 bronze badges
31110 bronze badges
New contributor
Abdelfettah Besbes is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Abdelfettah Besbes is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f57875417%2fdefining-a-function-which-returns-a-function-pointer-which-also-returns-a-functi%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
heSM7DbbyU9ahLKNWc4oZlC,cFlIst9aRtvAfSlz,7YOjqiujBP4vQamyFI,JH7,IJXlyHW5oQ pLV S4P3 JmjK E,gz3,CWbO6FZ382e
5
Good question - now don't ever do this again. :)
– Michael Dorgan
10 hours ago
5
use cdecl
– Antti Haapala
9 hours ago